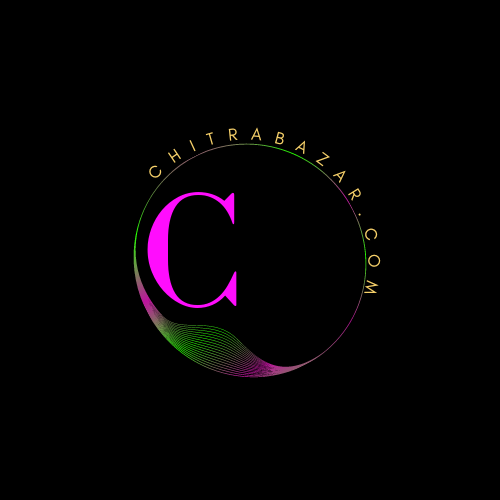
Working with iFrames
Introduction to iFrames
An inline frame, or iframe
, is an HTML element that allows embedding another HTML document within the current one. It is widely used for embedding videos, maps, ads, and other external content. Understanding how to work with iframes effectively can significantly enhance the functionality and interactivity of your web pages.
Basic Implementation
The simplest way to add an iframe to your webpage is using the <iframe>
tag. Here’s a basic example:
<iframe src="example.com" width="600" height="400"></iframe>
This code embeds a webpage from example.com into your page with a width of 600 pixels and a height of 400 pixels.
Attributes of the <iframe> Tag
Attribute | Description | Example |
---|---|---|
src |
Specifies the URL of the document to embed. | <iframe src="https://example.com"></iframe> |
width |
Sets the width of the iframe. | <iframe width="600"></iframe> |
height |
Sets the height of the iframe. | <iframe height="400"></iframe> |
frameborder |
Defines whether the iframe should have a border (1) or not (0). Deprecated in HTML5. | <iframe frameborder="0"></iframe> |
allowfullscreen |
Allows the iframe content to be displayed in fullscreen mode. | <iframe allowfullscreen></iframe> |
loading |
Indicates how the iframe should be loaded: eager or lazy . |
<iframe loading="lazy"></iframe> |
Customization and Styling
Styling iframes can be done through CSS. Here’s an example of how to style an iframe:
<style>
iframe {
border: 2px solid #000;
border-radius: 8px;
}
</style>
<iframe src="https://example.com" width="600" height="400"></iframe>
Responsive iFrames
Making iframes responsive involves setting their width and height to adapt to different screen sizes. Here’s an example using CSS:
<style>
.responsive-iframe {
position: relative;
width: 100%;
height: 0;
padding-bottom: 56.25%; /* 16:9 aspect ratio */
}
.responsive-iframe iframe {
position: absolute;
width: 100%;
height: 100%;
top: 0;
left: 0;
}
</style>
<div class="responsive-iframe">
<iframe src="https://example.com" frameborder="0" allowfullscreen></iframe>
</div>
Security Considerations
Embedding external content via iframes can introduce security risks. Here are some security attributes to mitigate these risks:
Attribute | Description | Example |
---|---|---|
sandbox |
Applies extra restrictions to the iframe content, such as disabling scripts and forms. | <iframe src="https://example.com" sandbox></iframe> |
allow |
Specifies a list of features that the iframe is allowed to use. | <iframe src="https://example.com" allow="geolocation; microphone; camera"></iframe> |
referrerpolicy |
Controls how much referrer information is sent when the iframe loads. | <iframe src="https://example.com" referrerpolicy="no-referrer"></iframe> |
SEO Considerations
Using iframes can impact SEO. Search engines may not index the content within iframes, which can affect your site’s visibility. Here are some best practices:
- Avoid overusing iframes; use them only when necessary.
- Provide alternative content or descriptions for the iframe content.
- Use the
title
attribute to describe the iframe’s content.
<iframe src="https://example.com" title="Example website" width="600" height="400"></iframe>
Advanced Techniques
Communicating with iFrames
PostMessage API allows communication between the parent page and the iframe. Here’s how you can send and receive messages:
// Parent page
var iframe = document.getElementById('myIframe');
iframe.contentWindow.postMessage('Hello from parent', 'https://example.com');
// iFrame content
window.addEventListener('message', function(event) {
if (event.origin === 'https://example.com') {
console.log('Message received:', event.data);
}
});
Dynamic iFrame Resizing
Adjusting the iframe size dynamically based on the content can improve the user experience. Here’s a simple way to do it:
// Parent page
function resizeIframe(iframe) {
iframe.style.height = iframe.contentWindow.document.body.scrollHeight + 'px';
}
// iFrame content
window.addEventListener('load', function() {
window.parent.postMessage({
type: 'resize',
height: document.body.scrollHeight
}, '*');
});
// Parent page - listen for resize messages
window.addEventListener('message', function(event) {
if (event.data.type === 'resize') {
var iframe = document.getElementById('myIframe');
iframe.style.height = event.data.height + 'px';
}
});
Common Issues and Troubleshooting
Here are some common issues when working with iframes and how to troubleshoot them:
- Content not displaying: Ensure the URL is correct and accessible. Check for CORS (Cross-Origin Resource Sharing) issues.
- Security errors: Review and adjust security-related attributes like
sandbox
andallow
. - Responsive design issues: Ensure proper CSS is applied for responsive iframes.
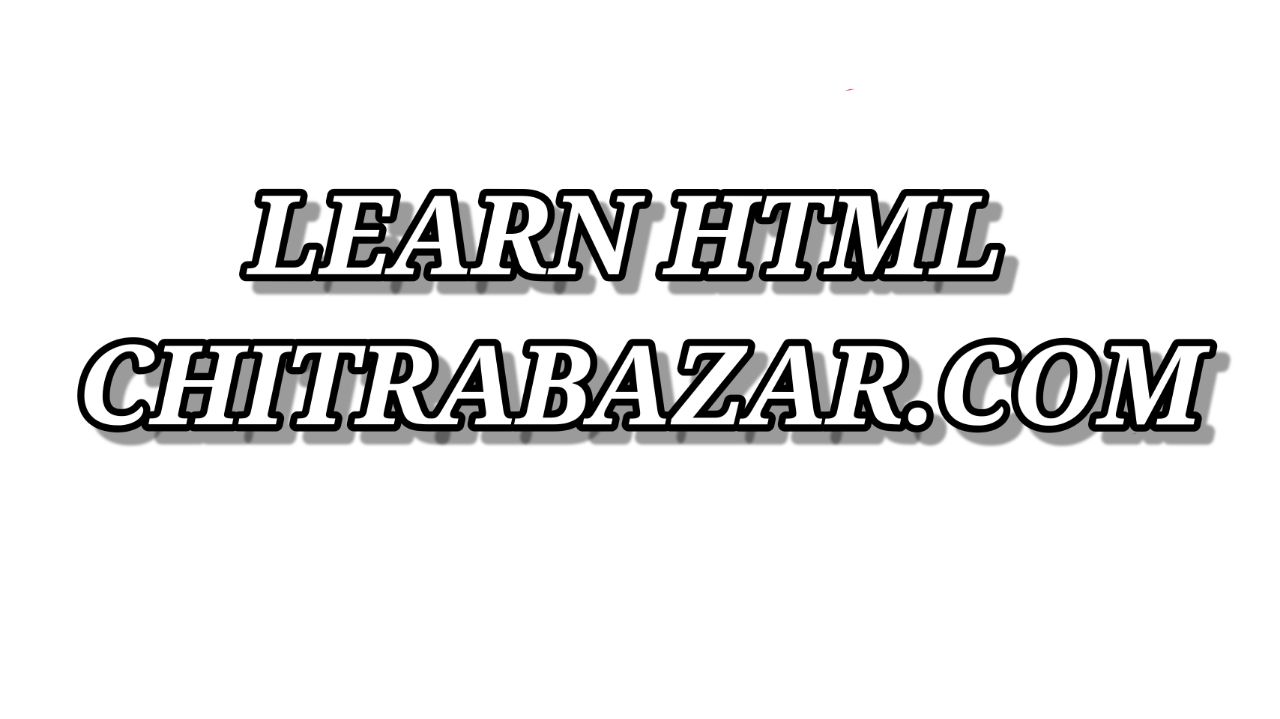