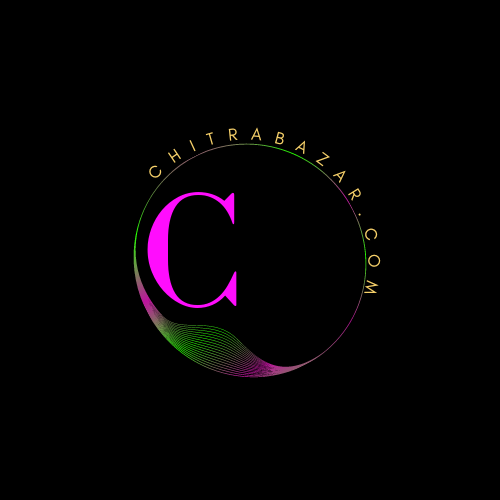
Radio Buttons and Checkboxes
Overview of Radio buttons and checkboxes
Radio buttons and checkboxes are integral components in web forms. They provide users with options to select from and can be used to gather various types of input. This guide covers the implementation, styling, and accessibility considerations for radio buttons and checkboxes.
Radio Buttons
Radio buttons allow users to select one option from a group of predefined options. Only one radio button in a group can be selected at a time.
Basic HTML Structure
Radio buttons are created using the <input>
element with the type attribute set to "radio". Each radio button in a group must share the same name
attribute to ensure only one can be selected at a time.
Example
<form>
<div class="form-group">
<label>Choose a color:</label>
<input type="radio" id="colorRed" name="color" value="red">
<label for="colorRed">Red</label>
<input type="radio" id="colorGreen" name="color" value="green">
<label for="colorGreen">Green</label>
<input type="radio" id="colorBlue" name="color" value="blue">
<label for="colorBlue">Blue</label>
</div>
</form>
In this example, three radio buttons are grouped under the name "color". Users can select only one color from the options.
Checkboxes
Checkboxes allow users to select multiple options from a group. Each checkbox is independent, meaning users can select any combination of checkboxes.
Basic HTML Structure
Checkboxes are created using the <input>
element with the type attribute set to "checkbox". Each checkbox can have a unique name
attribute, or they can share the same name
if their values are to be grouped.
Example
<form>
<div class="form-group">
<label>Select your hobbies:</label>
<input type="checkbox" id="hobbyReading" name="hobby" value="reading">
<label for="hobbyReading">Reading</label>
<input type="checkbox" id="hobbyTraveling" name="hobby" value="traveling">
<label for="hobbyTraveling">Traveling</label>
<input type="checkbox" id="hobbyCooking" name="hobby" value="cooking">
<label for="hobbyCooking">Cooking</label>
</div>
</form>
In this example, three checkboxes are provided for users to select their hobbies. Users can select one, two, or all three hobbies.
Styling Radio Buttons and Checkboxes
Radio buttons and checkboxes can be styled using CSS to improve their appearance and match the overall design of your website.
Example
<style>
input[type="radio"], input[type="checkbox"] {
display: none;
}
input[type="radio"] + label, input[type="checkbox"] + label {
position: relative;
padding-left: 25px;
cursor: pointer;
}
input[type="radio"] + label::before, input[type="checkbox"] + label::before {
content: '';
position: absolute;
left: 0;
top: 0;
width: 20px;
height: 20px;
border: 1px solid #ccc;
background-color: #fff;
}
input[type="radio"]:checked + label::before {
background-color: #66bb6a;
}
input[type="checkbox"]:checked + label::before {
background-color: #66bb6a;
}
</style>
<form>
<div class="form-group">
<label>Choose a color:</label>
<input type="radio" id="colorRed" name="color" value="red">
<label for="colorRed">Red</label>
<input type="radio" id="colorGreen" name="color" value="green">
<label for="colorGreen">Green</label>
<input type="radio" id="colorBlue" name="color" value="blue">
<label for="colorBlue">Blue</label>
</div>
<div class="form-group">
<label>Select your hobbies:</label>
<input type="checkbox" id="hobbyReading" name="hobby" value="reading">
<label for="hobbyReading">Reading</label>
<input type="checkbox" id="hobbyTraveling" name="hobby" value="traveling">
<label for="hobbyTraveling">Traveling</label>
<input type="checkbox" id="hobbyCooking" name="hobby" value="cooking">
<label for="hobbyCooking">Cooking</label>
</div>
</form>
This example hides the default radio buttons and checkboxes using display: none;
and styles the labels to create custom checkboxes and radio buttons.
Accessibility Considerations
Ensuring that radio buttons and checkboxes are accessible is crucial for users who rely on assistive technologies.
Using ARIA Attributes
ARIA (Accessible Rich Internet Applications) attributes can enhance the accessibility of radio buttons and checkboxes.
Example
<form>
<fieldset>
<legend>Choose a color:</legend>
<div class="form-group">
<input type="radio" id="colorRed" name="color" value="red" aria-labelledby="colorRedLabel">
<label id="colorRedLabel" for="colorRed">Red</label>
<input type="radio" id="colorGreen" name="color" value="green" aria-labelledby="colorGreenLabel">
<label id="colorGreenLabel" for="colorGreen">Green</label>
<input type="radio" id="colorBlue" name="color" value="blue" aria-labelledby="colorBlueLabel">
<label id="colorBlueLabel" for="colorBlue">Blue</label>
</div>
</fieldset>
<fieldset>
<legend>Select your hobbies:</legend>
<div class="form-group">
<input type="checkbox" id="hobbyReading" name="hobby" value="reading" aria-labelledby="hobbyReadingLabel">
<label id="hobbyReadingLabel" for="hobbyReading">Reading</label>
<input type="checkbox" id="hobbyTraveling" name="hobby" value="travel
ing" aria-labelledby="hobbyTravelingLabel">
<label id="hobbyTravelingLabel" for="hobbyTraveling">Traveling</label>
<input type="checkbox" id="hobbyCooking" name="hobby" value="cooking" aria-labelledby="hobbyCookingLabel">
<label id="hobbyCookingLabel" for="hobbyCooking">Cooking</label>
</div>
</fieldset>
</form>
This example uses the aria-labelledby
attribute to ensure that screen readers correctly associate the radio buttons and checkboxes with their corresponding labels.
Grouping Radio Buttons and Checkboxes
Grouping related radio buttons and checkboxes helps users understand the context and relationships between options.
Using Fieldsets and Legends
The <fieldset>
and <legend>
elements provide a way to group related form controls and give them a descriptive heading.
Example
<form>
<fieldset>
<legend>Personal Information</legend>
<div class="form-group">
<label>Gender:</label>
<input type="radio" id="genderMale" name="gender" value="male">
<label for="genderMale">Male</label>
<input type="radio" id="genderFemale" name="gender" value="female">
<label for="genderFemale">Female</label>
</div>
<div class="form-group">
<label>Interests:</label>
<input type="checkbox" id="interestMusic" name="interest" value="music">
<label for="interestMusic">Music</label>
<input type="checkbox" id="interestSports" name="interest" value="sports">
<label for="interestSports">Sports</label>
<input type="checkbox" id="interestMovies" name="interest" value="movies">
<label for="interestMovies">Movies</label>
</div>
</fieldset>
</form>
This example uses <fieldset>
and <legend>
to group gender radio buttons and interest checkboxes under the heading "Personal Information".
Advanced Features
Enhancing radio buttons and checkboxes with JavaScript can provide additional functionality and improve user experience.
Toggle All Checkboxes
You can create a "Select All" checkbox that toggles the state of all other checkboxes in a group.
Example
<form>
<div class="form-group">
<input type="checkbox" id="selectAll" name="selectAll">
<label for="selectAll">Select All</label>
</div>
<div class="form-group">
<input type="checkbox" id="option1" name="option" value="1">
<label for="option1">Option 1</label>
<input type="checkbox" id="option2" name="option" value="2">
<label for="option2">Option 2</label>
<input type="checkbox" id="option3" name="option" value="3">
<label for="option3">Option 3</label>
</div>
</form>
<script>
document.getElementById('selectAll').addEventListener('change', function() {
var checkboxes = document.querySelectorAll('input[name="option"]');
for (var checkbox of checkboxes) {
checkbox.checked = this.checked;
}
});
</script>
This example adds a "Select All" checkbox that toggles the state of all other checkboxes in the group. When "Select All" is checked, all checkboxes are selected; when it is unchecked, all checkboxes are deselected.
Validation
Validating radio buttons and checkboxes ensures that users provide the necessary input before submitting the form.
Example
<form onsubmit="return validateForm()">
<fieldset>
<legend>Survey</legend>
<div class="form-group">
<label>Do you like our website?</label>
<input type="radio" id="likeYes" name="like" value="yes">
<label for="likeYes">Yes</label>
<input type="radio" id="likeNo" name="like" value="no">
<label for="likeNo">No</label>
</div>
<div class="form-group">
<label>What features do you use?</label>
<input type="checkbox" id="feature1" name="feature" value="feature1">
<label for="feature1">Feature 1</label>
<input type="checkbox" id="feature2" name="feature" value="feature2">
<label for="feature2">Feature 2</label>
<input type="checkbox" id="feature3" name="feature" value="feature3">
<label for="feature3">Feature 3</label>
</div>
<button type="submit">Submit</button>
</fieldset>
</form>
<script>
function validateForm() {
var radios = document.getElementsByName('like');
var formValid = false;
var i = 0;
while (!formValid && i < radios.length) {
if (radios[i].checked) formValid = true;
i++;
}
if (!formValid) {
alert('Please select an option for "Do you like our website?"');
return false;
}
var checkboxes = document.getElementsByName('feature');
var checkedOne = Array.prototype.slice.call(checkboxes).some(x => x.checked);
if (!checkedOne) {
alert('Please select at least one feature.');
return false;
}
return true;
}
</script>
This example includes a validation function that checks if at least one radio button and one checkbox are selected before allowing the form to be submitted.
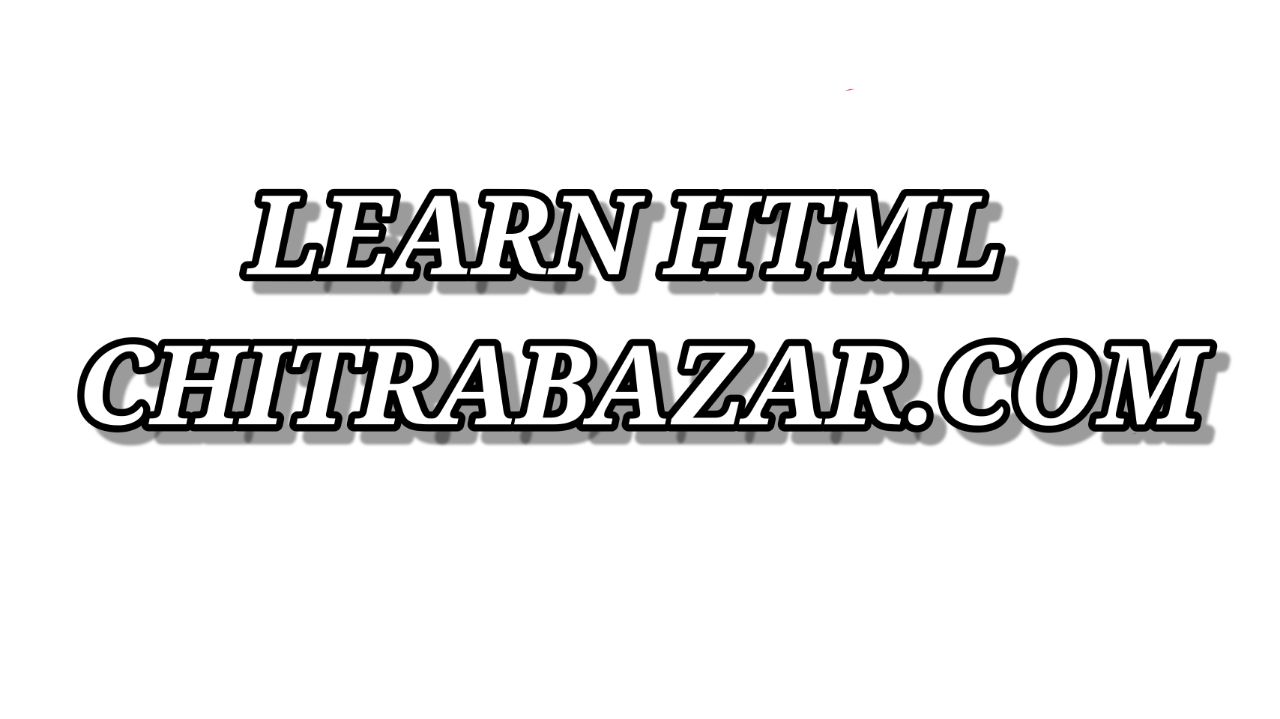