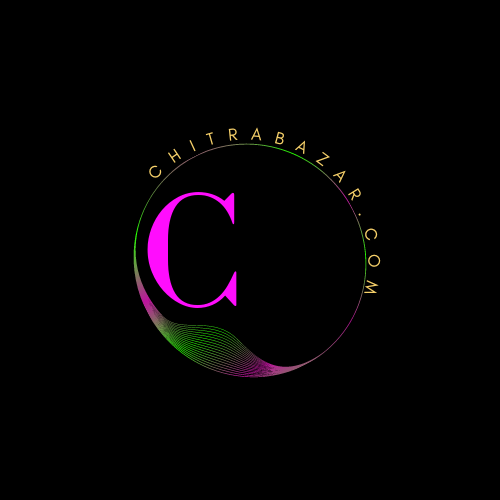
Audio and Video Embedding
Introduction to Embedding Media
Embedding audio and video in web pages enhances the user experience by providing dynamic content. This guide covers the technical details of embedding media, including HTML tags, attributes, and examples.
Audio Embedding
HTML5 Audio Tag
The HTML5 <audio>
tag is used to embed audio files. It supports various audio formats such as MP3, Ogg, and WAV. Below is the basic syntax for embedding an audio file:
<audio controls>
<source src="audiofile.mp3" type="audio/mpeg">
<source src="audiofile.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
Attributes of the <audio>
Tag
controls
: Adds play, pause, and volume controls.autoplay
: Automatically starts playing the audio as soon as it is loaded.loop
: Repeats the audio after it finishes.muted
: Mutes the audio by default.preload
: Specifies if and how the author thinks the audio should be loaded when the page loads. Possible values arenone
,metadata
, andauto
.
Example of Embedded Audio
<audio controls autoplay loop muted preload="auto">
<source src="song.mp3" type="audio/mpeg">
<source src="song.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
Video Embedding
HTML5 Video Tag
The HTML5 <video>
tag is used to embed video files. It supports formats like MP4, WebM, and Ogg. Here is the basic syntax for embedding a video file:
<video width="320" height="240" controls>
<source src="videofile.mp4" type="video/mp4">
<source src="videofile.webm" type="video/webm">
Your browser does not support the video element.
</video>
Attributes of the <video>
Tag
controls
: Adds play, pause, and volume controls.autoplay
: Automatically starts playing the video as soon as it is loaded.loop
: Repeats the video after it finishes.muted
: Mutes the video by default.preload
: Specifies if and how the author thinks the video should be loaded when the page loads. Possible values arenone
,metadata
, andauto
.width
andheight
: Specifies the width and height of the video player.poster
: Specifies an image to be shown while the video is downloading or until the user hits the play button.
Example of Embedded Video
<video width="640" height="480" controls autoplay loop muted preload="auto" poster="posterimage.jpg">
<source src="movie.mp4" type="video/mp4">
<source src="movie.webm" type="video/webm">
Your browser does not support the video element.
</video>
Embedding Videos from External Sources
Videos from platforms like YouTube and Vimeo can be embedded using their provided iframe embed codes.
Example of Embedding a YouTube Video
<iframe width="560" height="315" src="https://www.youtube.com/embed/dQw4w9WgXcQ" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
Example of Embedding a Vimeo Video
<iframe src="https://player.vimeo.com/video/123456789" width="640" height="360" frameborder="0" allow="autoplay; fullscreen; picture-in-picture" allowfullscreen></iframe>
Advanced Techniques
Using JavaScript for Enhanced Control
JavaScript can be used to control audio and video elements programmatically, offering advanced functionalities such as custom controls, event listeners, and more.
Example of JavaScript Control for Video
<video id="myVideo" width="320" height="240" controls>
<source src="videofile.mp4" type="video/mp4">
Your browser does not support the video element.
</video>
<button onclick="playPause()">Play/Pause</button>
<button onclick="makeBig()">Big</button>
<button onclick="makeSmall()">Small</button>
<button onclick="makeNormal()">Normal</button>
<script>
var vid = document.getElementById("myVideo");
function playPause() {
if (vid.paused) {
vid.play();
} else {
vid.pause();
}
}
function makeBig() {
vid.width = 560;
}
function makeSmall() {
vid.width = 320;
}
function makeNormal() {
vid.width = 420;
}
</script>
Responsive Embedding
Ensuring that embedded media is responsive is crucial for a seamless user experience across different devices and screen sizes.
Example of Responsive Video Embedding
<style>
.responsive-video {
position: relative;
padding-bottom: 56.25%;
height: 0;
overflow: hidden;
max-width: 100%;
background: #000;
}
.responsive-video iframe,
.responsive-video object,
.responsive-video embed {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>
<div class="responsive-video">
<iframe src="https://www.youtube.com/embed/vsTIRTyKZUw" frameborder="0" allowfullscreen></iframe>
</div>
Common Issues and Troubleshooting
Browser Compatibility
Different browsers support different audio and video formats. It's essential to include multiple formats to ensure compatibility.
Supported Formats by Browser
Browser | Audio Formats | Video Formats |
---|---|---|
Chrome | MP3, Ogg, WAV | MP4, WebM, Ogg |
Firefox | MP3, Ogg, WAV | MP4, WebM, Ogg |
Safari | MP3, WAV | MP4 |
Edge | MP3, Ogg, WAV | MP4, WebM, Ogg |
Internet Explorer | MP3, WAV | MP4 |
Handling Fallback Content
Fallback content is essential for users whose browsers do not support the <audio>
or <video>
tags. This can be done by providing a message or a link to download the media file.
Example of Fallback Content
<audio controls>
<source src="audiofile.mp3" type="audio/mpeg">
<source src="audiofile.ogg" type="audio/ogg">
Your browser does not support the audio element.
<a href="audiofile.mp3">Download the audio</a>
</audio>
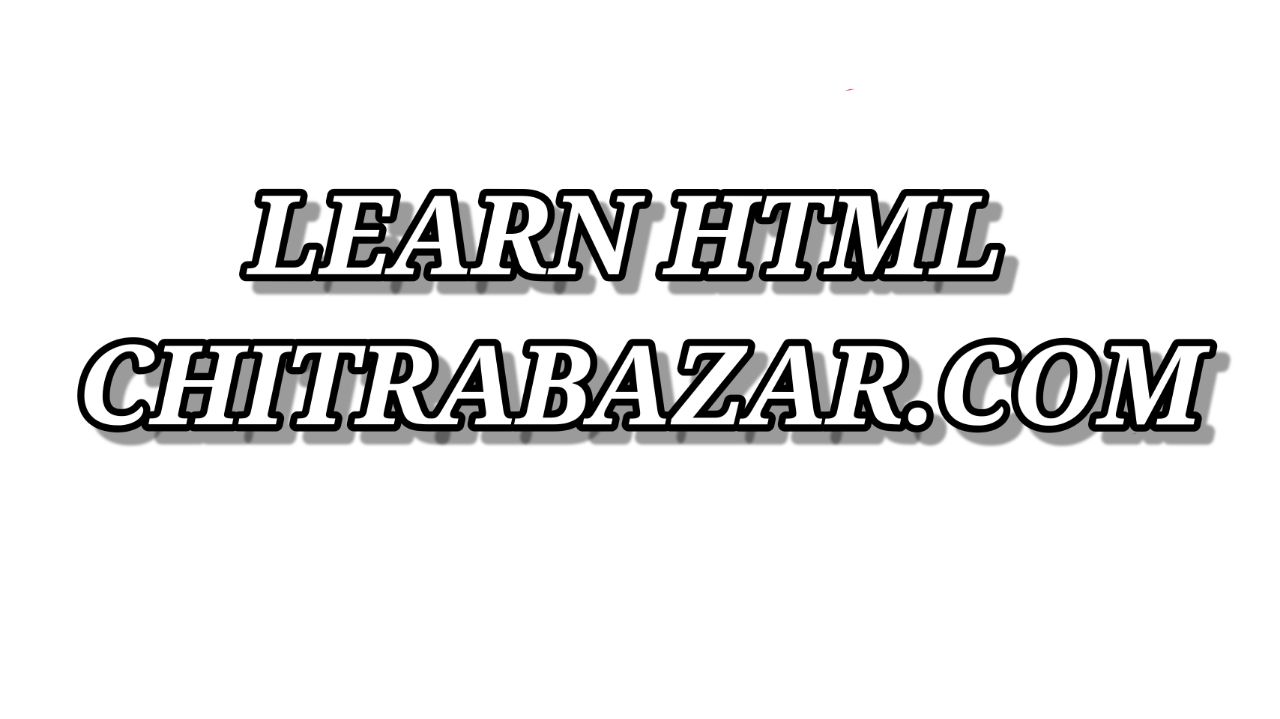
Audio and Video Embedding FAQ
Question 1: What is the basic syntax for embedding an audio file using HTML5?
Answer: The basic syntax for embedding an audio file using HTML5 is:
<audio controls>
<source src="audiofile.mp3" type="audio/mpeg">
<source src="audiofile.ogg" type="audio/ogg">
Your browser does not support the audio element.
</audio>
Question 2: Which attributes can be used with the <audio> tag?
Answer: The attributes that can be used with the <audio> tag are:
controls
autoplay
loop
muted
preload
Question 3: How do you embed a video file in HTML5?
Answer: To embed a video file in HTML5, you can use the following syntax:
<video width="320" height="240" controls>
<source src="videofile.mp4" type="video/mp4">
<source src="videofile.webm" type="video/webm">
Your browser does not support the video element.
</video>
Question 4: What is the purpose of the poster
attribute in the <video> tag?
Answer: The poster
attribute specifies an image to be shown while the video is downloading or until the user hits the play button.
Question 5: How can you embed a YouTube video on a webpage?
Answer: You can embed a YouTube video on a webpage using the following iframe code:
<iframe width="560" height="315" src="https://www.youtube.com/embed/dQw4w9WgXcQ" title="YouTube video player" frameborder="0" allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
Question 6: What are the possible values for the preload
attribute in the <audio> and <video> tags?
Answer: The possible values for the preload
attribute are:
none
metadata
auto
Question 7: How can you make an embedded video responsive?
Answer: To make an embedded video responsive, you can use the following CSS and HTML structure:
<style>
.responsive-video {
position: relative;
padding-bottom: 56.25%;
height: 0;
overflow: hidden;
max-width: 100%;
background: #000;
}
.responsive-video iframe,
.responsive-video object,
.responsive-video embed {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
</style>
<div class="responsive-video">
<iframe src="https://www.youtube.com/embed/dQw4w9WgXcQ" frameborder="0" allowfullscreen></iframe>
</div>
Question 8: What is the role of JavaScript in controlling video elements?
Answer: JavaScript can be used to control video elements programmatically, offering advanced functionalities such as custom controls and event listeners.
Question 9: What should you do if a user's browser does not support the <audio> or <video> tags?
Answer: If a user's browser does not support the <audio> or <video> tags, you should provide fallback content such as a message or a link to download the media file. Example:
<audio controls>
<source src="audiofile.mp3" type="audio/mpeg">
<source src="audiofile.ogg" type="audio/ogg">
Your browser does not support the audio element.
<a href="audiofile.mp3">Download the audio</a>
</audio>
Question 10: Which audio and video formats are supported by major browsers?
Answer: The supported formats by major browsers are:
Browser | Audio Formats | Video Formats |
---|---|---|
Chrome | MP3, Ogg, WAV | MP4, WebM, Ogg |
Firefox | MP3, Ogg, WAV | MP4, WebM, Ogg |
Safari | MP3, WAV | MP4 |
Edge | MP3, Ogg, WAV | MP4, WebM, Ogg |
Internet Explorer | MP3, WAV | MP4 |