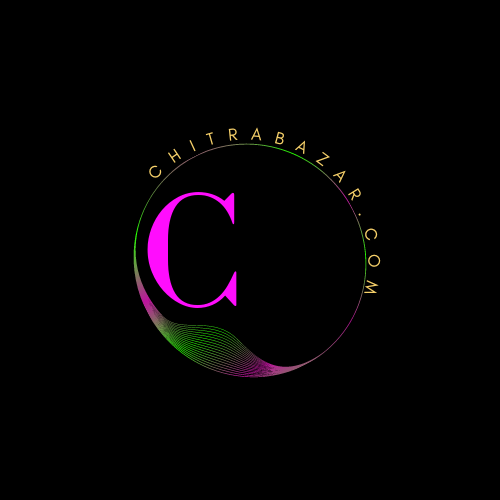
Building a Responsive Web Page
Introduction of Building a Responsive Web Page
A responsive web page adapts to different screen sizes and devices, providing an optimal user experience regardless of whether the user is on a desktop, tablet, or mobile phone. This guide covers the essential concepts and techniques for building a responsive web page, including fluid grids, flexible images, and media queries.
Fluid Grids
A fluid grid layout uses relative units like percentages instead of fixed units like pixels. This allows the layout to adjust dynamically to different screen sizes.
Example of a Fluid Grid
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Fluid Grid Example</title>
<style>
.container {
width: 100%;
max-width: 1200px;
margin: auto;
padding: 1em;
}
.row {
display: flex;
flex-wrap: wrap;
}
.col {
flex: 1;
padding: 1em;
box-sizing: border-box;
}
</style>
</head>
<body>
<div class="container">
<div class="row">
<div class="col" style="flex: 1 1 50%;">Column 1</div>
<div class="col" style="flex: 1 1 50%;">Column 2</div>
</div>
</div>
</body>
</html>
Using CSS Flexbox
CSS Flexbox is a powerful layout module that allows for the creation of flexible and responsive layouts. Flexbox makes it easy to align elements horizontally and vertically, distribute space, and manage layout changes dynamically.
Example of Flexbox Layout
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexbox Example</title>
<style>
.container {
display: flex;
flex-wrap: wrap;
margin: -10px;
}
.item {
flex: 1;
padding: 10px;
box-sizing: border-box;
background: #f4f4f4;
margin: 10px;
}
</style>
</head>
<body>
<div class="container">
<div class="item">Item 1</div>
<div class="item">Item 2</div>
<div class="item">Item 3</div>
</div>
</body>
</html>
Flexible Images
Flexible images adjust to the size of their container, ensuring they do not overflow and maintain their aspect ratio. This can be achieved using CSS properties like max-width
and height: auto
.
Example of Flexible Images
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Flexible Images Example</title>
<style>
img {
max-width: 100%;
height: auto;
}
</style>
</head>
<body>
<div>
<img src="example.jpg" alt="Example Image">
</div>
</body>
</html>
Media Queries
Media queries allow you to apply CSS rules based on the characteristics of the device, such as its width, height, orientation, and resolution. This is a cornerstone of responsive design, enabling the creation of layouts that adapt to different screen sizes.
Example of Media Queries
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Media Queries Example</title>
<style>
.container {
width: 100%;
padding: 1em;
box-sizing: border-box;
}
.col {
float: left;
width: 100%;
}
@media(min-width: 600px) {
.col {
width: 50%;
}
}
@media(min-width: 768px) {
.col {
width: 33.33%;
}
}
</style>
</head>
<body>
<div class="container">
<div class="col">Column 1</div>
<div class="col">Column 2</div>
<div class="col">Column 3</div>
</div>
</body>
</html>
Responsive Navigation
Responsive navigation menus adjust to the screen size and provide an easy-to-use interface on both desktop and mobile devices. This can be achieved using CSS and JavaScript .
Example of Responsive Navigation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navigation Example</title>
<style>
body {
font-family: Arial, sans-serif;
}
.navbar {
display: flex;
justify-content: space-between;
background: #333;
padding: 1em;
}
.navbar a {
color: #fff;
text-decoration: none;
padding: 0.5em 1em;
}
.navbar a:hover {
background: #575757;
}
.navbar .menu {
display: none;
}
@media (max-width: 768px) {
.navbar {
flex-direction: column;
align-items: flex-start;
}
.navbar .menu {
display: block;
}
}
</style>
</head>
<body>
<div class="navbar">
<a href="#" class="brand">Brand</a>
<a href="#" class="menu">Menu</a>
<div class="links">
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Services</a>
<a href="#">Contact</a>
</div>
</div>
</body>
</html>
Responsive Typography
Responsive typography ensures that text is legible on all devices. This can be achieved using relative units like em
and rem
, and media queries to adjust font sizes based on screen width.
Example of Responsive Typography
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Typography Example</title>
<style>
body {
font-size: 16px;
}
h1 {
font-size: 2em;
}
p {
font-size: 1em;
}
@media (max-width: 768px) {
body {
font-size: 14px;
}
h1 {
font-size: 1.5em;
}
p {
font-size: 0.875em;
}
}
</style>
</head>
<body>
<h1>Responsive Typography Example</h1>
<p>This is a paragraph with responsive typography. Resize the browser window to see the text size adjust accordingly.</p>
</body>
</html>
Responsive Images
Responsive images adjust to the size of their container, ensuring they do not overflow and maintain their aspect ratio. This can be achieved using CSS properties like max-width
and height: auto
, and the srcset
attribute in HTML to provide different image sources for different resolutions.
Example of Responsive Images
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Images Example</title>
<style>
img {
max-width: 100%;
height: auto;
}
</style>
</head>
<body>
<div>
<img src="example.jpg" srcset="example-300.jpg 300w, example-600.jpg 600w, example-1200.jpg 1200w" alt="Example Image">
</div>
</body>
</html>
Responsive Frameworks
Responsive frameworks like Bootstrap and Foundation provide pre-built CSS and JavaScript components that help developers quickly create responsive web pages. These frameworks include grid systems, responsive utilities, and various UI components.
Example of Using Bootstrap
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Bootstrap Example</title>
<link rel="stylesheet" href="#">
</head>
<body>
<div class="container">
<div class="row">
<div class="col-md-4">Column 1</div>
<div class="col-md-4">Column 2</div>
<div class="col-md-4">Column 3</div>
</div>
</div>
<script src="#"></script>
<script src="#"></script>
<script src="#"></script>
</body>
</html>
Testing and Debugging
Testing and debugging are crucial for ensuring that a responsive web page works as intended across different devices and browsers. Tools like Chrome DevTools, responsive design mode in Firefox, and online services like BrowserStack can help test and debug responsive designs.
Using Chrome DevTools
Chrome DevTools provides a device toolbar that allows you to simulate different screen sizes and resolutions. You can access this by opening DevTools (F12 or Ctrl+Shift+I) and clicking on the device toolbar icon.
Example of Chrome DevTools
<img src="chrome-devtools.png" alt="Chrome DevTools">
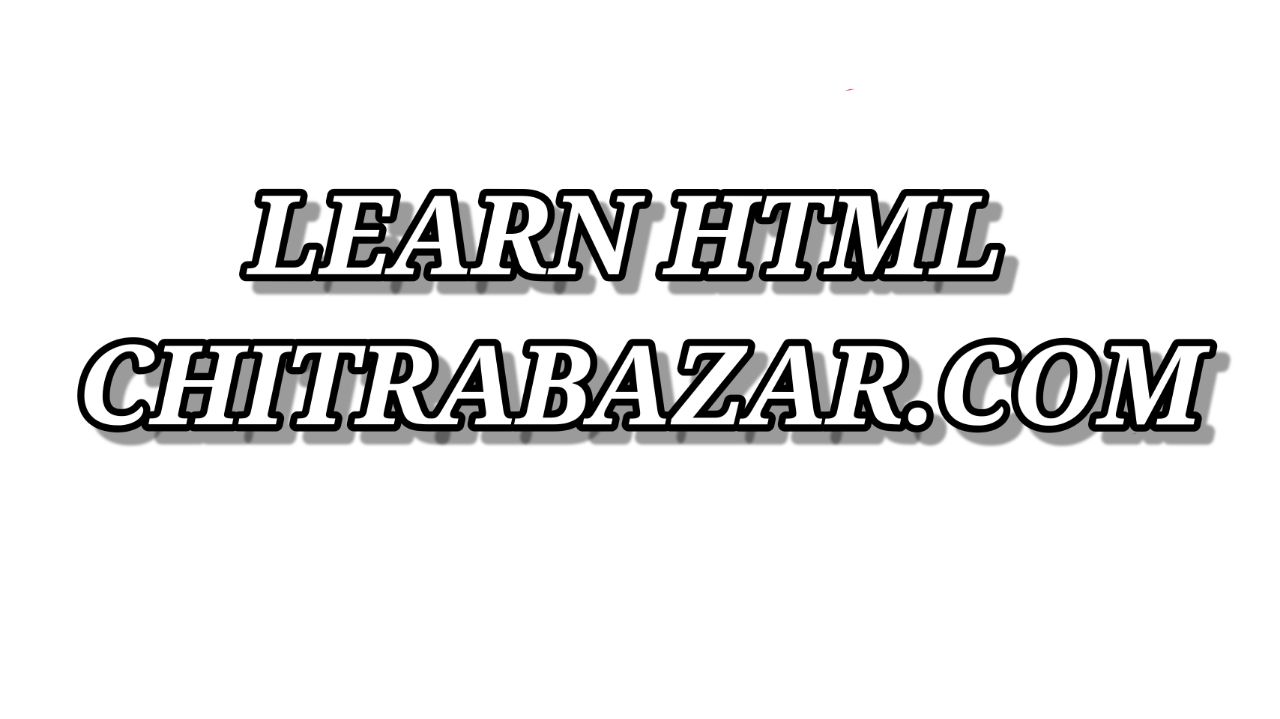
Responsive Web Page FAQ
1. What is a fluid grid in web design?
A fluid grid layout uses relative units like percentages instead of fixed units like pixels. This allows the layout to adjust dynamically to different screen sizes.
2. How does CSS Flexbox help in creating responsive layouts?
CSS Flexbox is a layout module that allows for the creation of flexible and responsive layouts. It makes it easy to align elements horizontally and vertically, distribute space, and manage layout changes dynamically.
3. What CSS properties are used to make images flexible?
CSS properties like max-width: 100%;
and height: auto;
are used to make images flexible. This ensures images adjust to the size of their container and maintain their aspect ratio.
4. What are media queries and how are they used?
Media queries are CSS rules that apply styles based on the characteristics of the device, such as its width, height, orientation, and resolution. They enable the creation of layouts that adapt to different screen sizes.
5. How can you create a responsive navigation menu?
A responsive navigation menu can be created using CSS and JavaScript. It involves using media queries to adjust the menu's appearance on different screen sizes and potentially using JavaScript to toggle the menu visibility on smaller screens.
6. What is the purpose of using relative units like em
and rem
for typography?
Using relative units like em
and rem
for typography ensures that text sizes adjust proportionally to the base font size, making the text more scalable and responsive across different devices.
7. What is the srcset
attribute in HTML and how is it used?
The srcset
attribute in HTML is used to define multiple image sources for different resolutions. It helps in providing responsive images that adjust based on the device's screen size and resolution.
8. What are some popular responsive frameworks?
Some popular responsive frameworks include Bootstrap and Foundation. These frameworks provide pre-built CSS and JavaScript components that help developers quickly create responsive web pages.
9. How can you test and debug responsive web designs?
Responsive web designs can be tested and debugged using tools like Chrome DevTools, responsive design mode in Firefox, and online services like BrowserStack. These tools allow you to simulate different screen sizes and resolutions.
10. Why is responsive design important?
Responsive design is important because it ensures that web pages provide an optimal user experience across different devices and screen sizes. It helps in improving usability, accessibility, and overall user satisfaction.