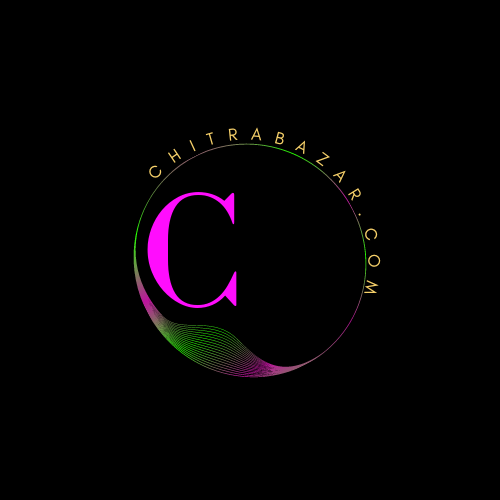
Organizing Content with Divs
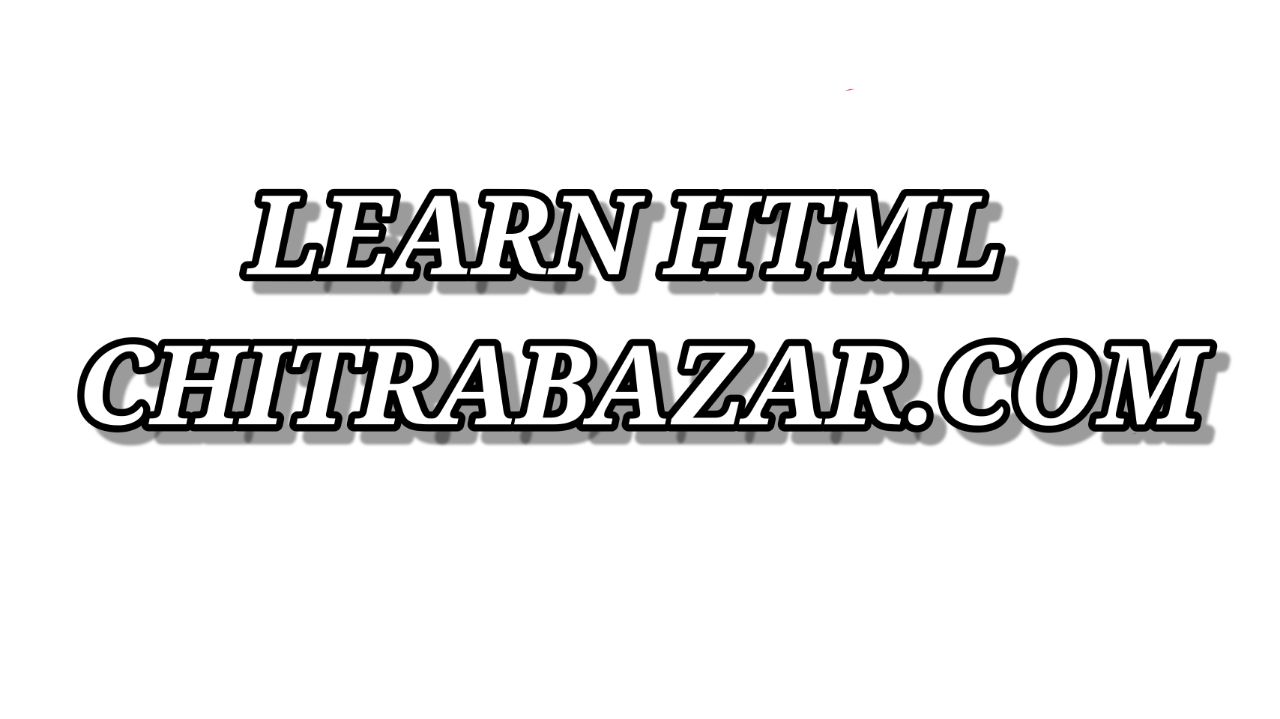
Organizing Content with Divs
Main Content Area
Using divs, you can create a well-structured layout for your website. The div element is a block-level container that can hold various types of content and can be styled with CSS to create complex layouts. Here are some techniques to organize content using divs:
Basic Structure
Start with a simple structure by using divs to create sections of your page:
<div class="container">
<div class="header">...</div>
<div class="content">
<div class="main">...</div>
<div class="sidebar">...</div>
</div>
<div class="footer">...</div>
</div>
Flexbox Layout
Flexbox is a powerful layout module that allows you to design flexible and efficient layouts:
.content {
display: flex;
flex-direction: row;
}
.main {
flex: 3;
}
.sidebar {
flex: 1;
}
This configuration creates a main content area that takes up three times the space of the sidebar.
Grid Layout
CSS Grid Layout provides a two-dimensional layout system, which is ideal for dividing a page into major regions:
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 10px;
}
.grid-item {
padding: 20px;
}
Example of a grid layout:
Nested Divs
Divs can be nested to create more complex structures. This can help in organizing content hierarchically:
<div class="parent">
<div class="child">...</div>
<div class="child">...</div>
</div>
Example:
Sidebar
Sidebars can be used for additional navigation or information:
- Links
- Ads
- Related Articles
Footer Content
Example of Flexbox Layout
Example of Grid Layout
Organizing Content with Divs - FAQ
<div class="container">
<div class="header">Header</div>
<div class="content">
<div class="main">Main Content</div>
<div class="sidebar">Sidebar</div>
</div>
<div class="footer">Footer</div>
</div>
div {
background-color: #f4f4f4;
padding: 20px;
}
.container {
width: 80%;
margin: 0 auto;
}
.container {
display: flex;
}
.main {
flex: 3;
}
.sidebar {
flex: 1;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 10px;
}
@media (max-width: 600px) {
.container {
flex-direction: column;
}
}
<div class="parent">
<div class="child">Child 1</div>
<div class="child">Child 2</div>
</div>
.container {
display: flex;
justify-content: center;
align-items: center;
}
.clearfix::after {
content: "";
clear: both;
display: table;
}
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
.scrollable {
overflow: auto;
}
div {
padding: 20px;
margin: 10px;
}