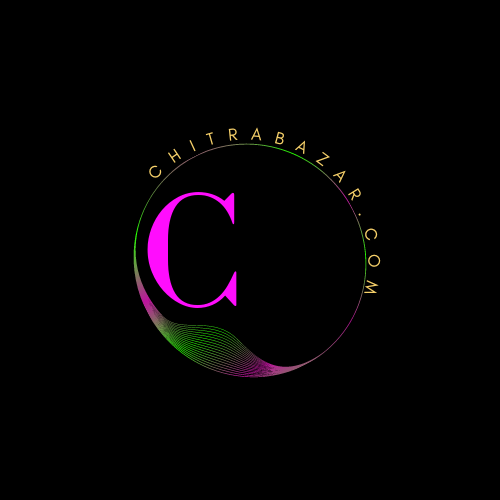
Embedding Google Maps
Learn about How to Embedding Google Maps
Embedding Google Maps into your website can enhance the user experience by providing interactive maps that show locations, directions, and other geographical data. This guide will explain how to embed Google Maps using HTML and JavaScript, covering various methods and customization options.
Basic Embedding using an Iframe
The simplest way to embed a Google Map is by using an iframe. This method does not require any programming knowledge and is straightforward to implement:
<iframe
width="600"
height="450"
style="border:0"
loading="lazy"
allowfullscreen
src="https://www.google.com/maps/embed/v1/place?key=YOUR_API_KEY&q=Place+Name,City,Country">
</iframe>
Replace YOUR_API_KEY
with your actual Google Maps API key, and Place+Name,City,Country
with the desired location.
Getting a Google Maps API Key
To embed maps with more advanced features, you need a Google Maps API key. Follow these steps to obtain one:
- Go to the Google Maps Platform.
- Click on "Get Started" and select the products you want to use (e.g., Maps, Routes, Places).
- Create a new project and set up billing (note: Google offers a free tier with some usage limitations).
- Once your project is created, go to the APIs & Services > Credentials page.
- Click "Create credentials" and select "API key".
- Copy the generated API key and keep it secure.
Embedding a Customized Google Map
To embed a customized Google Map, you can use the Google Maps JavaScript API. This method provides more flexibility and customization options:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Customized Google Map</title>
<style>
#map {
height: 450px;
width: 100%;
}
</style>
</head>
<body>
<h2>My Customized Google Map</h2>
<div id="map"></div>
<script>
function initMap() {
var location = { lat: -25.344, lng: 131.036 };
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: location
});
var marker = new google.maps.Marker({
position: location,
map: map
});
}
</script>
<script src="#YOUR_API_KEY" async defer></script>
</body>
</html>
In this example, replace YOUR_API_KEY
with your actual API key. The initMap
function initializes the map, sets its center and zoom level, and adds a marker.
Adding Multiple Markers
To add multiple markers to your map, you can modify the initMap
function to include an array of locations and loop through them to create markers:
<script>
function initMap() {
var locations = [
{ lat: -25.344, lng: 131.036 },
{ lat: -26.344, lng: 132.036 },
{ lat: -27.344, lng: 133.036 }
];
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: locations[0]
});
for (var i = 0; i < locations.length; i++) {
var marker = new google.maps.Marker({
position: locations[i],
map: map
});
}
}
</script>
Customizing Map Styles
Google Maps API allows you to customize the map's appearance using the styles
property. You can create a unique look for your map by defining style rules:
<script>
function initMap() {
var location = { lat: -25.344, lng: 131.036 };
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: location,
styles: [
{ elementType: 'geometry', stylers: [{ color: '#242f3e' }] },
{ elementType: 'labels.text.stroke', stylers: [{ color: '#242f3e' }] },
{ elementType: 'labels.text.fill', stylers: [{ color: '#746855' }] },
{
featureType: 'administrative.locality',
elementType: 'labels.text.fill',
stylers: [{ color: '#d59563' }]
},
{
featureType: 'poi',
elementType: 'labels.text.fill',
stylers: [{ color: '#d59563' }]
},
{
featureType: 'poi.park',
elementType: 'geometry',
stylers: [{ color: '#263c3f' }]
},
{
featureType: 'poi.park',
elementType: 'labels.text.fill',
stylers: [{ color: '#6b9a76' }]
},
{
featureType: 'road',
elementType: 'geometry',
stylers: [{ color: '#38414e' }]
},
{
featureType: 'road',
elementType: 'geometry.stroke',
stylers: [{ color: '#212a37' }]
},
{
featureType: 'road',
elementType: 'labels.text.fill',
stylers: [{ color: '#9ca5b3' }]
},
{
featureType: 'road.highway',
elementType: 'geometry',
stylers: [{ color: '#746855' }]
},
{
featureType: 'road.highway',
elementType: 'geometry.stroke',
stylers: [{ color: '#1f2835' }]
},
{
featureType: 'road.highway',
elementType: 'labels.text.fill',
stylers: [{ color: '#f3d19c' }]
},
{
featureType: 'transit',
elementType: 'geometry',
stylers: [{ color: '#2f3948' }]
},
{
featureType: 'transit.station',
elementType: 'labels.text.fill',
stylers: [{ color: '#d59563' }]
},
{
featureType: 'water',
elementType: 'geometry',
stylers: [{ color: '#17263c' }]
},
{
featureType: 'water',
elementType: 'labels.text.fill',
stylers: [{ color: '#515c6d' }]
},
{
featureType: 'water',
elementType: 'labels.text.stroke',
stylers: [{ color: '#17263c' }]
}
]
});
var marker = new google.maps.Marker({
position: location,
map: map
});
}
</script>
Adding Info Windows
Info windows display information when the user clicks on a marker. To add an info window, modify the initMap
function:
<script>
function initMap() {
var location = { lat: -25.344, lng: 131.036 };
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: location
});
var marker = new google.maps.Marker({
position: location,
map: map
});
var infowindow = new google.maps.InfoWindow({
content: '<h3>Uluru</h3><p>A large sandstone rock formation.</p>'
});
marker.addListener('click', function() {
infowindow.open(map, marker);
});
}
</script>
Handling Geolocation
You can use the browser's geolocation API to center the map on the user's current location. This requires user permission:
<script>
function initMap() {
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 6,
center: { lat: -25.344, lng: 131.036 }
});
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(function(position) {
var pos = {
lat: position.coords.latitude,
lng: position.coords.longitude
};
map.setCenter(pos);
var marker = new google.maps.Marker({
position: pos,
map: map
});
}, function() {
handleLocationError(true, map.getCenter());
});
} else {
handleLocationError(false, map.getCenter());
}
}
function handleLocationError(browserHasGeolocation, pos) {
var infoWindow = new google.maps.InfoWindow({
position: pos,
content: browserHasGeolocation ?
'Error: The Geolocation service failed.' :
'Error: Your browser doesn\'t support geolocation.'
});
infoWindow.open(map);
}
</script>
Embedding Google Maps with Directions
To embed Google Maps with directions, you can use the DirectionsService and DirectionsRenderer classes. This allows you to display a route between two or more points:
<script>
function initMap() {
var directionsService = new google.maps.DirectionsService();
var directionsRenderer = new google.maps.DirectionsRenderer();
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 7,
center: { lat: 41.85, lng: -87.65 }
});
directionsRenderer.setMap(map);
var request = {
origin: 'Chicago, IL',
destination: 'Los Angeles, CA',
travelMode: 'DRIVING'
};
directionsService.route(request, function(result, status) {
if (status == 'OK') {
directionsRenderer.setDirections(result);
}
});
}
</script>
Handling Errors and Limits
Google Maps API has usage limits and may return errors if these limits are exceeded. You should handle errors gracefully to ensure a good user experience:
<script>
function initMap() {
var location = { lat: -25.344, lng: 131.036 };
var map = new google.maps.Map(document.getElementById('map'), {
zoom: 4,
center: location
});
var marker = new google.maps.Marker({
position: location,
map: map
});
var geocoder = new google.maps.Geocoder();
geocodeAddress(geocoder, map);
function geocodeAddress(geocoder, resultsMap) {
var address = 'Sydney, NSW';
geocoder.geocode({ 'address': address }, function(results, status) {
if (status === 'OK') {
resultsMap.setCenter(results[0].geometry.location);
var marker = new google.maps.Marker({
map: resultsMap,
position: results[0].geometry.location
});
} else {
alert('Geocode was not successful for the following reason: ' + status);
}
});
}
}
</script>
Frequently Asked Questions about Embedding Google Maps
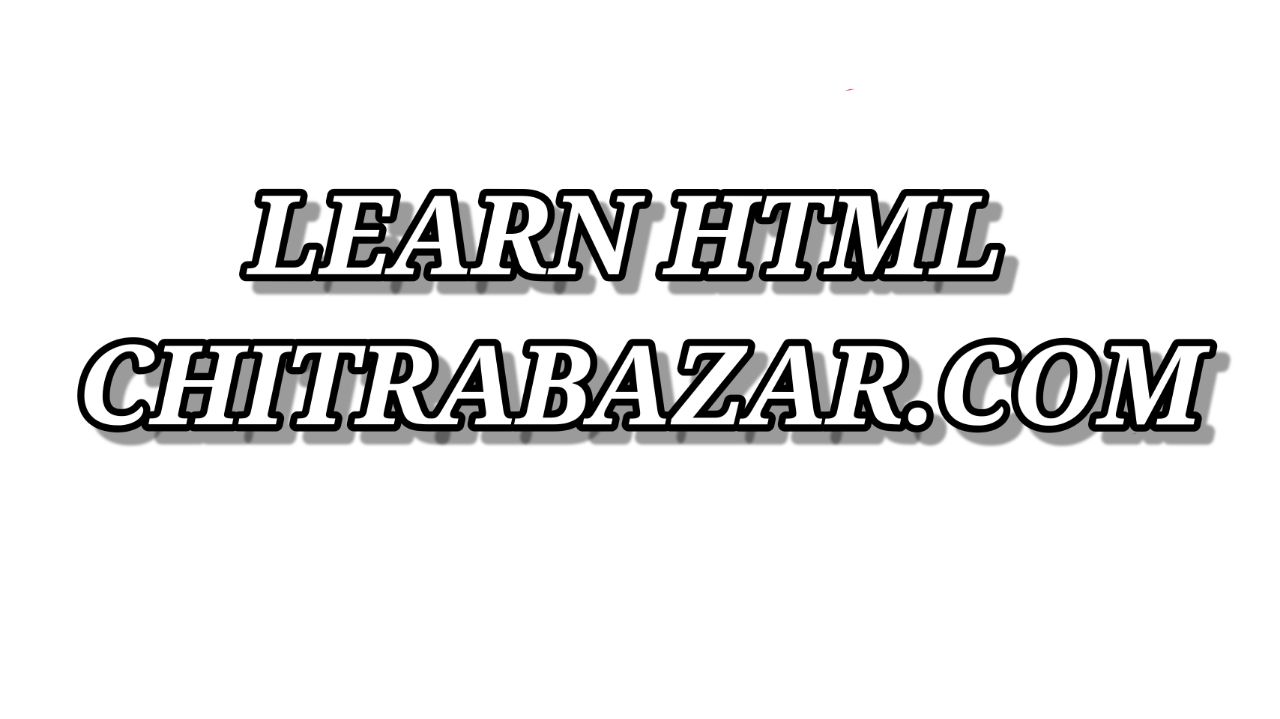