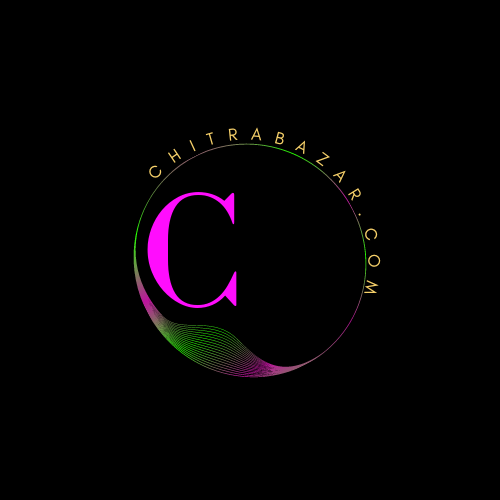
Working with HTML Forms
Working with HTML Forms
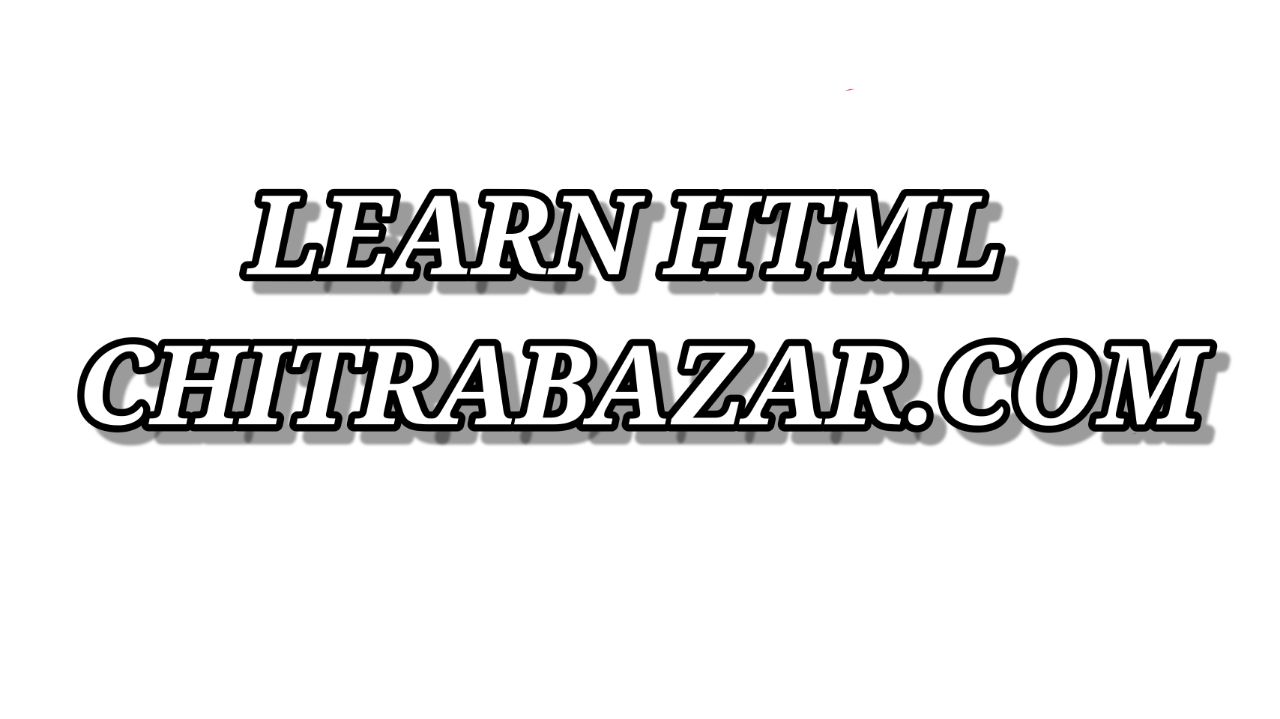
HTML forms are a crucial part of web development. They enable users to interact with web applications by submitting data. Whether it's logging in, signing up, or just leaving feedback, forms are everywhere on the web. This article provides a comprehensive guide to working with HTML forms, covering everything from basic syntax to advanced features.
Basic Structure of an HTML Form
An HTML form is defined using the <form>
element. This element contains input elements where users can enter data. Here's a simple example:
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br>
<input type="submit" value="Submit">
</form>
In this example:
- The
action
attribute specifies where to send the form data when the form is submitted. - The
method
attribute specifies the HTTP method to use when sending form data. Common methods areGET
andPOST
. - The
<label>
element defines a label for an<input>
element, improving accessibility. - The
<input>
element represents a field where users can enter data.
Types of Input Elements
HTML provides various input types, each suited for different kinds of data. Some common input types include:
text
: A single-line text field.password
: A single-line text field that masks the input.email
: A single-line text field for entering email addresses, with basic validation.number
: A single-line text field for entering numbers.checkbox
: A checkbox input.radio
: A radio button input.submit
: A button to submit the form.reset
: A button to reset the form fields to their default values.
Here is an example demonstrating some of these input types:
<form action="submit.php" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br>
<label for="age">Age:</label>
<input type="number" id="age" name="age"><br>
<input type="checkbox" id="subscribe" name="subscribe">
<label for="subscribe">Subscribe to newsletter</label><br>
<input type="radio" id="male" name="gender" value="male">
<label for="male">Male</label><br>
<input type="radio" id="female" name="gender" value="female">
<label for="female">Female</label><br>
<input type="submit" value="Submit">
<input type="reset" value="Reset">
</form>
Form Validation
Form validation ensures that the data submitted by the user meets specific criteria. HTML5 introduced several built-in validation features that make this easier.
Required Fields
To make a field required, add the required
attribute:
<label for="username">Username:</label>
<input type="text" id="username" name="username" required><br>
Pattern Matching
The pattern
attribute specifies a regular expression that the input's value must match:
<label for="username">Username:</label>
<input type="text" id="username" name="username" pattern="[A-Za-z]{3,}" title="Only letters, at least 3 characters"><br>
Other Validation Attributes
min
andmax
: Define the minimum and maximum values for number inputs.minlength
andmaxlength
: Define the minimum and maximum number of characters for text inputs.step
: Specifies the legal number intervals for number inputs.
Grouping Form Elements
Grouping form elements can improve the organization and usability of your forms. HTML provides the <fieldset>
and <legend>
elements for this purpose:
<form action="submit.php" method="post">
<fieldset>
<legend>Personal Information</legend>
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br>
</fieldset>
<fieldset>
<legend>Login Details</legend>
<label for="username">Username:</label>
<input type="text" id="username" name="username"><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br>
</fieldset>
<input type="submit" value="Submit">
</form>
Accessibility Considerations
Ensuring that forms are accessible is crucial. Here are some best practices:
- Use
<label>
elements with thefor
attribute to associate labels with their corresponding input fields. - Use the
<fieldset>
and<legend>
elements to group related form elements. - Provide meaningful and descriptive text for form controls.
- Ensure that form controls are keyboard navigable.
- Use ARIA (Accessible Rich Internet Applications) attributes to enhance accessibility where necessary.
Styling Forms
Styling forms can improve the user experience and make forms more visually appealing. CSS is used to style form elements. Here are some tips for styling forms:
- Use CSS to create a consistent layout and spacing between form elements.
- Style input fields to match the overall design of your site.
- Provide visual feedback for focus and hover states on form controls.
- Use CSS transitions to create smooth interactions.
Here's an example of a styled form:
<style>
form {
max-width: 400px;
margin: auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 10px;
background-color: #f9f9f9;
}
label {
display: block;
margin-bottom: 10px;
color: #333;
}
input[type="text"],
input[type="email"],
input[type="password"],
input[type="number"] {
width: 100%;
padding: 8px;
margin-bottom: 10px;
border: 1px solid #ccc;
border-radius: 5px;
}
input[type="submit"],
input[type="reset"] {
padding: 10px 15px;
border: none;
border-radius: 5px;
background-color: #28a745;
color: #fff;
cursor: pointer;
}
input[type="submit"]:hover,
input[type="reset"]:hover {
background-color: #218838;
}
</style>
<form action="submit.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name"><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email"><br>
<label for="password">Password:</label>
<input type="password" id="password" name="password"><br>
<input type="submit" value="Submit">
<input type="reset" value="Reset">
</form>
Handling Form Submissions
When a form is submitted, the data is sent to the server specified in the action
attribute of the form. The server then processes the data. Here’s a basic example of handling form submissions using PHP:
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$name = htmlspecialchars($_POST['name']);
$email = htmlspecialchars($_POST['email']);
$password = htmlspecialchars($_POST['password']);
echo "Name: $name<br>";
echo "Email: $email<br>";
echo "Password: $password<br>";
}
?>
This PHP script:
- Checks if the form was submitted using the POST method.
- Retrieves and sanitizes the form data using the
htmlspecialchars
function. - Displays the sanitized data.
Advanced Form Features
HTML forms support various advanced features that enhance functionality and user experience. Here are a few:
File Uploads
To allow users to upload files, use the file
input type:
<form action="upload.php" method="post" enctype="multipart/form-data">
<label for="file">Upload a file:</label>
<input type="file" id="file" name="file"><br>
<input type="submit" value="Upload">
</form>
The enctype="multipart/form-data"
attribute is necessary for file uploads.
Autofocus and Placeholder
The autofocus
attribute automatically focuses an input field when the page loads, and the placeholder
attribute provides a hint to the user:
<label for="username">Username:</label>
<input type="text" id="username" name="username" placeholder="Enter your username" autofocus><br>
Datalist
The datalist
element provides a list of predefined options for an input field:
<label for="browsers">Choose your browser:</label>
<input list="browsers" name="browser" id="browser">
<datalist id="browsers">
<option value="Chrome">
<option value="Firefox">
<option value="Safari">
<option value="Edge">
<option value="Opera">
</datalist>