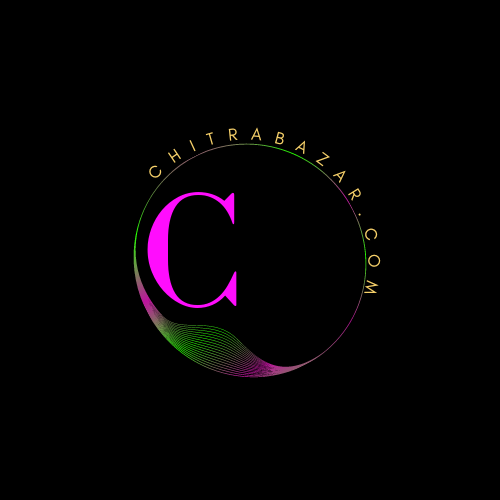
Audio and Video Controls
Audio and Video Controls in HTML
Introduction
HTML5 introduced the <audio>
and <video>
elements, providing native support for embedding audio and video content in web pages. These elements come with built-in controls that allow users to play, pause, and navigate media content. Understanding how to use and customize these controls can greatly enhance the multimedia experience on your website.
Basic Implementation
The <audio>
and <video>
elements are straightforward to implement. Here are basic examples:
Audio Element
<audio controls>
<source src="audiofile.mp3" type="audio/mpeg">
Your browser does not support the audio element.
</audio>
Video Element
<video controls width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
Your browser does not support the video element.
</video>
Attributes of Audio and Video Elements
Attribute | Description | Example |
---|---|---|
src |
Specifies the URL of the media file. | <audio src="audiofile.mp3" controls></audio> |
controls |
Displays built-in playback controls. | <video controls></video> |
autoplay |
Automatically starts playing the media as soon as it is loaded. | <audio autoplay></audio> |
loop |
Plays the media repeatedly. | <video loop></video> |
muted |
Mutes the audio output. | <audio muted></audio> |
preload |
Specifies if and how the author thinks the media should be loaded when the page loads. | <audio preload="auto"></audio> |
width |
Sets the width of the video player. | <video width="600"></video> |
height |
Sets the height of the video player. | <video height="400"></video> |
poster |
Specifies an image to be shown while the video is downloading or until the user hits the play button. | <video poster="posterimage.jpg"></video> |
Customizing Controls
Although the default controls are useful, you can create custom controls for a more tailored user experience. This requires some JavaScript. Here’s an example of custom controls for a video element:
HTML Structure
<div class="video-container">
<video id="customVideo" width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
</video>
<div class="controls">
<button onclick="playPause()">Play/Pause</button>
<button onclick="stopVideo()">Stop</button>
<input type="range" id="seekBar" value="0" step="0.01" max="1" onchange="seekVideo()">
</div>
</div>
CSS Styling
<style>
.video-container {
position: relative;
width: 600px;
}
.controls {
position: absolute;
bottom: 10px;
left: 0;
width: 100%;
display: flex;
justify-content: space-around;
background: rgba(0, 0, 0, 0.5);
padding: 10px;
}
.controls button, .controls input {
background: #fff;
border: none;
padding: 5px;
cursor: pointer;
}
</style>
JavaScript Functions
<script>
var video = document.getElementById('customVideo');
var seekBar = document.getElementById('seekBar');
function playPause() {
if (video.paused) {
video.play();
} else {
video.pause();
}
}
function stopVideo() {
video.pause();
video.currentTime = 0;
}
function seekVideo() {
var time = video.duration * (seekBar.value / 1);
video.currentTime = time;
}
video.addEventListener('timeupdate', function() {
var value = (video.currentTime / video.duration) * 1;
seekBar.value = value;
});
</script>
Accessibility Considerations
Ensuring your audio and video content is accessible to all users is crucial. Here are some tips to enhance accessibility:
- Provide captions and subtitles for video content.
- Use the
<track>
element to add text tracks for captions, subtitles, and descriptions. - Ensure keyboard accessibility for custom controls.
- Provide transcripts for audio content.
Adding Captions and Subtitles
<video controls width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
<track src="subtitles_en.vtt" kind="subtitles" srclang="en" label="English">
</video>
Best Practices for Audio and Video Controls
Follow these best practices to ensure a seamless multimedia experience:
- Use appropriate file formats and codecs for wide compatibility (e.g., MP4, WebM, Ogg for video; MP3, Ogg, WAV for audio).
- Optimize media files to reduce loading times and improve performance.
- Test media playback across different browsers and devices.
- Provide fallback content for unsupported browsers.
- Keep the user experience in mind when designing custom controls.
Advanced Techniques
Picture-in-Picture (PiP)
Picture-in-Picture (PiP) allows users to watch video in a small window that stays on top of other windows. Here’s how to enable PiP in your video player:
<video id="pipVideo" controls width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
</video>
<button onclick="togglePiP()">Toggle PiP</button>
<script>
var video = document.getElementById('pipVideo');
async function togglePiP() {
if (video !== document.pictureInPictureElement) {
try {
await video.requestPictureInPicture();
} catch (error) {
console.error('Error enabling PiP:', error);
}
} else {
try {
await document.exitPictureInPicture();
} catch (error) {
console.error('Error disabling PiP:', error);
}
}
}
</script>
Handling Multiple Audio Tracks
Sometimes, you may need to provide multiple audio tracks for different languages or commentary. Here’s how to handle multiple audio tracks:
<video controls width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
<track src="audiotrack1.vtt" kind="captions" srclang="en" label="English Audio">
<track src="audiotrack2.vtt" kind="captions" srclang="es" label="Spanish Audio">
</video>
Customizing Playback Speed
Allowing users to adjust the playback speed can enhance their viewing experience. Here’s an example:
<video id="speedVideo" controls width="600" height="400">
<source src="videofile.mp4" type="video/mp4">
</video>
<button onclick="setPlaybackSpeed(0.5)">0.5x</button>
<button onclick="setPlaybackSpeed(1)">1x</button>
<button onclick="setPlaybackSpeed(1.5)">1.5x</button>
<button onclick="setPlaybackSpeed(2)">2x</button>
<script>
var video = document.getElementById('speedVideo');
function setPlaybackSpeed(speed) {
video.playbackRate = speed;
}
</script>
Common Issues and Troubleshooting
Here are some common issues with audio and video controls and how to troubleshoot them:
- Media not playing: Check the file format and codec compatibility. Ensure the file path is correct.
- No sound: Verify that the audio is not muted and the volume is up. Check the audio track.
- Custom controls not working: Ensure your JavaScript functions are correctly linked and executed. Debug using browser developer tools.
- Accessibility issues: Ensure captions, subtitles, and transcripts are provided. Check keyboard accessibility for custom controls.
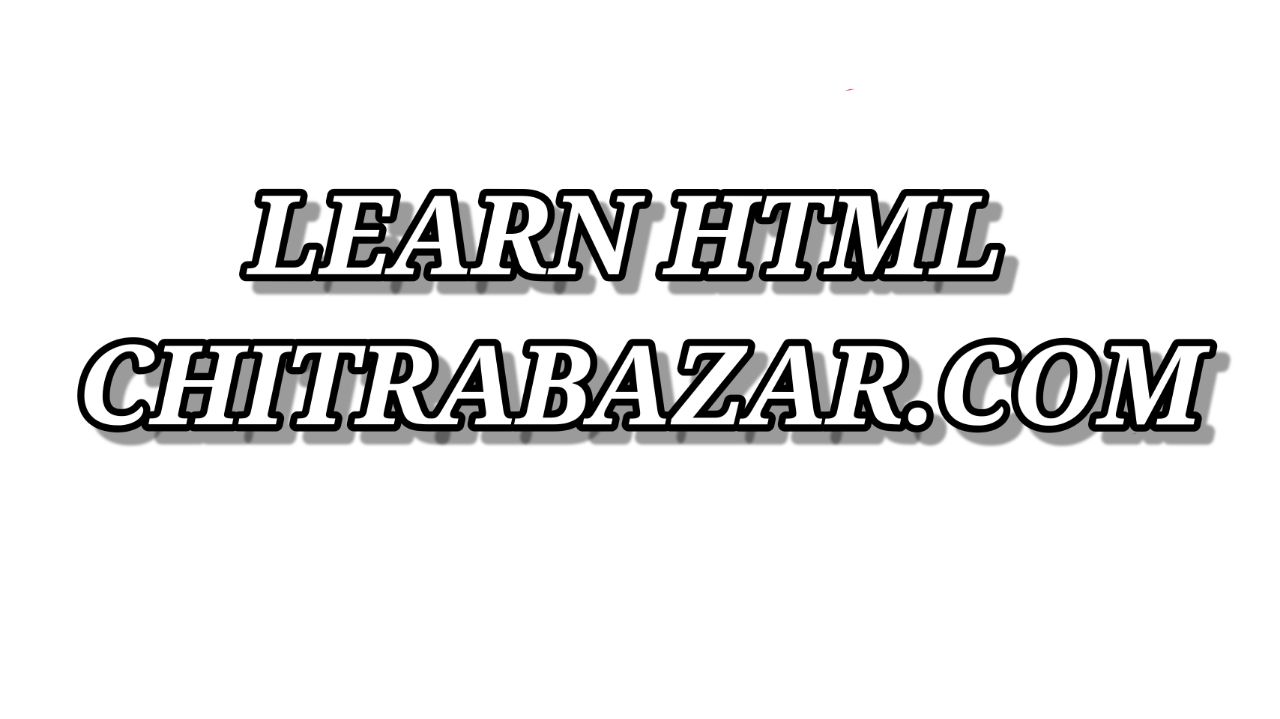