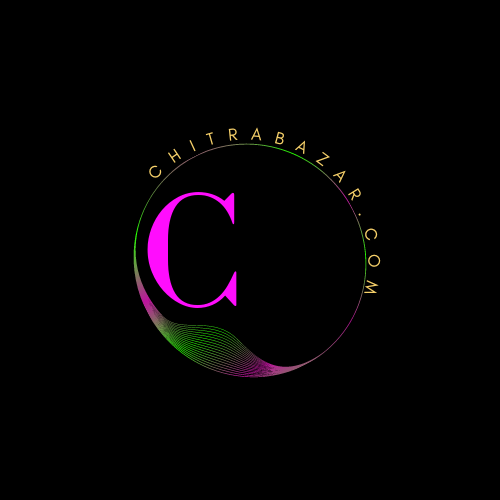
Building a Basic Web Page
Introduction of Web Page
Creating a basic web page is the first step towards understanding web development. This guide will walk you through the process of building a simple web page using HTML, the foundational language of the web. We will cover the basic structure, essential elements, styling, and best practices to ensure your web page is well-formed and functional.
HTML Structure
HTML (HyperText Markup Language) uses a series of elements to structure and display content on the web. Every HTML document starts with a doctype declaration, followed by the <html>
element that contains the <head>
and <body>
sections.
Basic HTML Document Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My First Web Page</title>
</head>
<body>
<h1>Welcome to My Web Page</h1>
<p>This is a paragraph of text on my first web page.</p>
</body>
</html>
Essential HTML Elements
HTML provides a variety of elements to structure content, including headings, paragraphs, links, images, and lists. Below are some of the most commonly used elements:
Headings
Headings are used to define the structure of your content, with <h1>
being the highest level and <h6>
the lowest.
<h1>Main Heading</h1>
<h2>Subheading</h2>
<h3>Sub-subheading</h3>
Paragraphs
Paragraphs are used to group blocks of text.
<p>This is a paragraph of text.</p>
Links
Links are created using the <a>
element, which requires an href
attribute to specify the URL.
<a href="https://www.example.com">Visit Example</a>
Images
Images are embedded using the <img>
element, which requires a src
attribute to specify the image file and an alt
attribute for alternative text.
<img src="image.jpg" alt="Description of image">
Lists
Lists can be ordered (<ol>
) or unordered (<ul>
), with each item in the list enclosed in a <li>
element.
<ul>
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
<ol>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ol>
Styling Your Web Page with CSS
CSS (Cascading Style Sheets) is used to style HTML elements. You can include CSS directly within the HTML document using the <style>
element or link to an external CSS file.
Internal CSS
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
color: #333;
}
p {
color: #555;
}
</style>
External CSS
<head>
<link rel="stylesheet" href="styles.css">
</head>
Basic CSS Properties
Here are some common CSS properties to style your web page:
Property | Description | Example |
---|---|---|
color |
Sets the text color. | color: #333; |
font-size |
Sets the size of the text. | font-size: 16px; |
margin |
Sets the space around elements. | margin: 20px; |
padding |
Sets the space inside elements. | padding: 10px; |
background-color |
Sets the background color of an element. | background-color: #f4f4f4; |
border |
Sets the border around an element. | border: 1px solid #ddd; |
Adding Interactivity with JavaScript
JavaScript is a scripting language used to create dynamic content on web pages. You can include JavaScript within the HTML document using the <script>
element or link to an external JavaScript file.
Internal JavaScript
<script>
function showAlert() {
alert('Hello, world!');
}
</script>
External JavaScript
<head>
<script src="script.js"></script>
</head>
Basic JavaScript Example
Here’s an example of a simple JavaScript function that changes the content of a paragraph when a button is clicked:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Example</title>
<script>
function changeText() {
document.getElementById('myParagraph').innerText = 'The text has been changed!';
}
</script>
</head>
<body>
<h1>JavaScript Example</h1>
<p id="myParagraph">This is a paragraph of text.</p>
<button onclick="changeText()">Change Text</button>
</body>
</html>
Best Practices for Building a Web Page
Following best practices ensures your web page is accessible, efficient, and maintainable:
- Use semantic HTML to improve accessibility and SEO (e.g.,
<header>
,<nav>
,<main>
,<footer>
). - Keep your CSS and JavaScript in separate files to enhance readability and maintainability.
- Optimize images for faster loading times.
- Ensure your web page is responsive and works well on all devices and screen sizes.
- Validate your HTML and CSS to catch errors and ensure compliance with web standards.
Responsive Design
Responsive design ensures your web page looks good on all devices. Use CSS media queries to apply different styles based on the device’s screen size.
Example of a Media Query
<style>
body {
font-family: Arial, sans-serif;
}
.container {
width: 100%;
max-width: 1200px;
margin: 0 auto;
}
@media (max-width: 600px) {
.container {
padding: 0 20px;
}
}
</style>
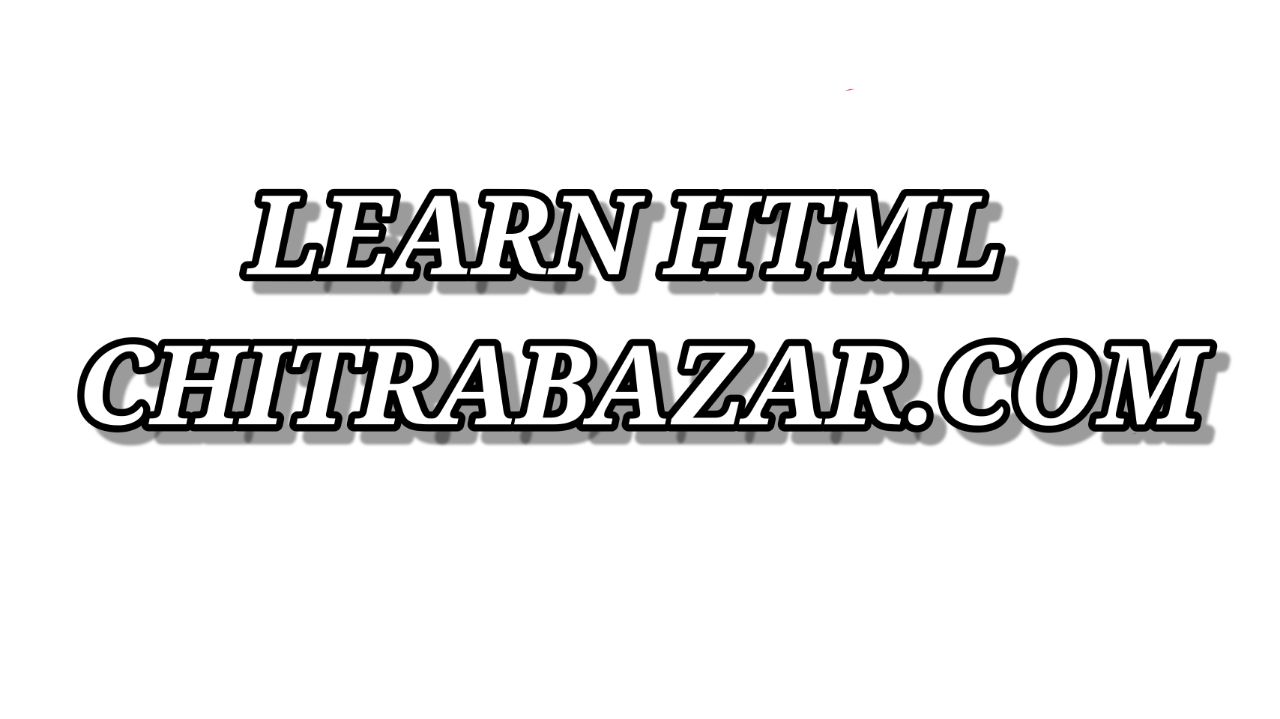