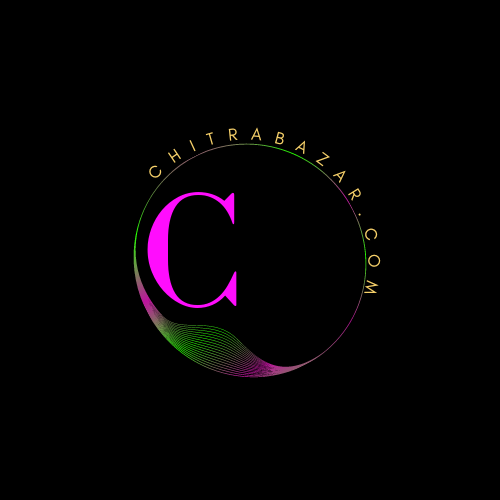
Creating a Navigation Bar
Learn about - How to Creating a Navigation Bar
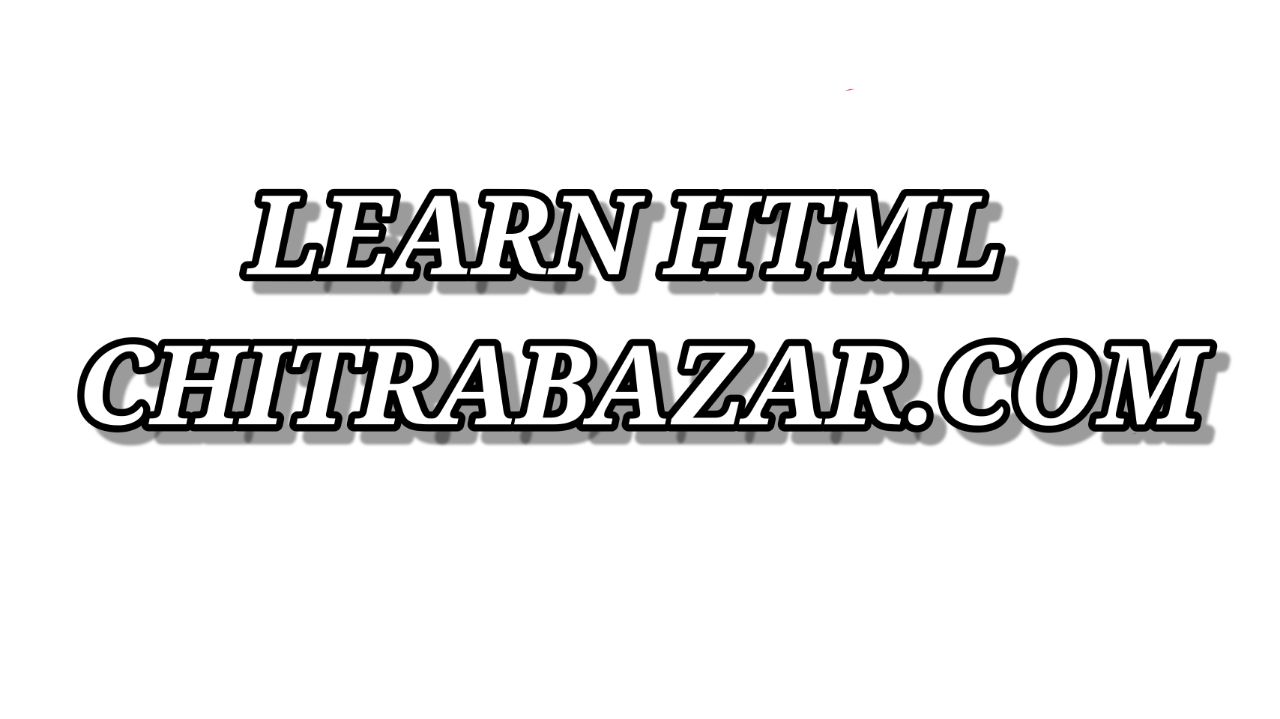
A navigation bar (navbar) is a graphical user interface element that allows users to navigate through a website. It typically contains links to the main sections of the website and is usually placed at the top of the page. Below, we'll explore how to create a responsive and functional navigation bar using HTML and CSS.
Basic Structure
To start, we need to create the basic structure of the navigation bar using HTML. This includes defining the navigation container and the individual links:
<nav>
<a href="#home">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
Styling the Navigation Bar
Next, we will style the navigation bar using CSS to make it visually appealing and functional:
nav {
background-color: #333;
overflow: hidden;
}
nav a {
float: left;
display: block;
color: #f2f2f2;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav a:hover {
background-color: #ddd;
color: black;
}
Responsive Design
To make the navigation bar responsive, we can use media queries to adjust the layout based on the screen size. For example, we can stack the links vertically on smaller screens:
@media screen and (max-width: 600px) {
nav a {
float: none;
display: block;
text-align: left;
}
}
Dropdown Menu
Adding a dropdown menu allows for more complex navigation structures. We can create a dropdown menu using a combination of HTML and CSS:
<nav>
<a href="#home">Home</a>
<a href="#services" class="dropdown">Services</a>
<div class="submenu">
<a href="#webdesign">Web Design</a>
<a href="#seo">SEO</a>
</div>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
.submenu {
display: none;
position: absolute;
background-color: #333;
min-width: 160px;
z-index: 1;
}
.submenu a {
float: none;
padding: 12px 16px;
text-decoration: none;
display: block;
text-align: left;
}
.dropdown:hover .submenu {
display: block;
}
JavaScript Enhancements
For more advanced interactions, you can use JavaScript to enhance the functionality of the navigation bar. For example, you can add a toggle button for the dropdown menu on smaller screens:
<nav>
<a href="#home">Home</a>
<a href="#services" class="dropdown">Services</a>
<div class="submenu">
<a href="#webdesign">Web Design</a>
<a href="#seo">SEO</a>
</div>
<a href="#about">About</a>
<a href="#contact">Contact</a>
<a href="javascript:void(0);" class="icon" onclick="toggleMenu()">☰</a>
</nav>
function toggleMenu() {
var x = document.querySelector("nav");
if (x.className === "") {
x.className += "responsive";
} else {
x.className = "";
}
}
Accessibility Considerations
It's important to ensure that the navigation bar is accessible to all users. This includes using semantic HTML elements and providing keyboard navigation support:
<nav role="navigation">
<a href="#home">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
Example: Full Navigation Bar Implementation
Below is a complete example of a responsive navigation bar with a dropdown menu and JavaScript enhancements:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Navigation Bar</title>
<style>
body {
font-family: Arial, sans-serif;
line-height: 1.6;
}
nav {
background-color: #333;
overflow: hidden;
}
nav a {
float: left;
display: block;
color: #f2f2f2;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav a:hover {
background-color: #ddd;
color: black;
}
.submenu {
display: none;
position: absolute;
background-color: #333;
min-width: 160px;
z-index: 1;
}
.submenu a {
float: none;
padding: 12px 16px;
text-decoration: none;
display: block;
text-align: left;
}
.submenu a:hover {
background-color: #ddd;
color: black;
}
.dropdown:hover .submenu {
display: block;
}
.icon {
display: none;
}
@media screen and (max-width: 600px) {
nav a:not(:first-child) {display: none;}
nav a.icon {
float: right;
display: block;
}
}
@media screen and (max-width: 600px) {
nav.responsive {position: relative;}
nav.responsive .icon {
position: absolute;
right: 0;
top: 0;
}
nav.responsive a {
float: none;
display: block;
text-align: left;
}
nav.responsive .submenu {position: relative;}
}
</style>
</head>
<body>
<nav>
<a href="#home">Home</a>
<a href="#services" class="dropdown">Services</a>
<div class="submenu">
<a href="#webdesign">Web Design</a>
<a href="#seo">SEO</a>
</div>
<a href="#about">About</a>
<a href="#contact">Contact</a>
<a href="javascript:void(0);" class="icon" onclick="toggleMenu()">☰</a>
</nav>
<script>
function toggleMenu() {
var x = document.querySelector("nav");
if (x.className === "") {
x.className += "responsive";
} else {
x.className = "";
}
}
</script>
</body>
</html>
FAQ - Creating a Navigation Bar
<nav>
<a href="#home">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
nav {
background-color: #333;
overflow: hidden;
}
nav a {
float: left;
display: block;
color: #f2f2f2;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
nav a:hover {
background-color: #ddd;
color: black;
}
@media screen and (max-width: 600px) {
nav a {
float: none;
display: block;
text-align: left;
}
}
<nav>
<a href="#home">Home</a>
<a href="#services" class="dropdown">Services</a>
<div class="submenu">
<a href="#webdesign">Web Design</a>
<a href="#seo">SEO</a>
</div>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
.submenu {
display: none;
position: absolute;
background-color: #333;
min-width: 160px;
z-index: 1;
}
.dropdown:hover .submenu {
display: block;
}
<nav>
<a href="#home">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
<a href="javascript:void(0);" class="icon" onclick="toggleMenu()">☰</a>
</nav>
<script>
function toggleMenu() {
var x = document.querySelector("nav");
if (x.className === "") {
x.className += "responsive";
} else {
x.className = "";
}
}
</script>
<nav role="navigation">
<a href="#home">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
nav {
display: flex;
justify-content: center;
background-color: #333;
overflow: hidden;
}
nav a {
color: #f2f2f2;
padding: 14px 16px;
text-decoration: none;
}
nav a:hover {
background-color: #ddd;
color: black;
}
<nav>
<a href="#home" class="active">Home</a>
<a href="#services">Services</a>
<a href="#about">About</a>
<a href="#contact">Contact</a>
</nav>
.active {
background-color: #4CAF50;
color: white;
}
<link rel="stylesheet" href="https://cdnjs
.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css">
<nav>
<a href="#home"><i class="fas fa-home"></i> Home</a>
<a href="#services"><i class="fas fa-concierge-bell"></i> Services</a>
<a href="#about"><i class="fas fa-info-circle"></i> About</a>
<a href="#contact"><i class="fas fa-envelope"></i> Contact</a>
</nav>
nav {
position: -webkit-sticky; /* For Safari */
position: sticky;
top: 0;
background-color: #333;
z-index: 1000;
}
nav a {
transition: background-color 0.3s, color 0.3s;
}
nav a:hover {
background-color: #ddd;
color: black;
}