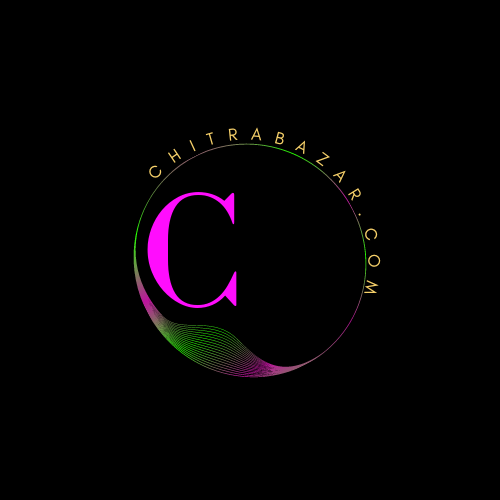
Creating Tooltips
Tooltips are small pop-up boxes that appear when the user hovers over an element. They provide additional information or context about the element without cluttering the interface. Tooltips can be implemented using various techniques in HTML, CSS, and JavaScript.
Basic Tooltip with HTML and CSS
A basic tooltip can be created using HTML and CSS without any JavaScript. The following example demonstrates a simple tooltip implementation:
<div class="tooltip">
Hover over me
<span class="tooltiptext">Tooltip text</span>
</div>
In this example, the tooltip text is placed inside a <span>
element with the class tooltiptext
. The tooltip is hidden by default and made visible when the user hovers over the parent element:
<style>
.tooltip {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip .tooltiptext {
visibility: hidden;
width: 120px;
background-color: black;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 5px 0;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
margin-left: -60px;
opacity: 0;
transition: opacity 0.3s;
}
.tooltip:hover .tooltiptext {
visibility: visible;
opacity: 1;
}
</style>
This CSS code styles the tooltip and handles its visibility. The .tooltip
class is applied to the parent element, and the .tooltiptext
class is applied to the tooltip itself. The tooltip is initially hidden using visibility: hidden
and opacity: 0
. When the parent element is hovered over, the tooltip becomes visible with visibility: visible
and opacity: 1
, thanks to the :hover
selector.
Advanced Tooltip with JavaScript
While the basic tooltip implementation is functional, adding JavaScript can provide greater control and flexibility. For example, you can dynamically create tooltips, control their position, and add interactive content. The following example demonstrates a more advanced tooltip implementation using JavaScript:
<style>
.tooltip-advanced {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip-advanced .tooltiptext-advanced {
visibility: hidden;
width: 120px;
background-color: black;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 5px 0;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
margin-left: -60px;
opacity: 0;
transition: opacity 0.3s;
}
</style>
<div class="tooltip-advanced" data-tooltip="Dynamic tooltip text">
Hover over me
</div>
<script>
document.addEventListener('DOMContentLoaded', function() {
var tooltipElements = document.querySelectorAll('.tooltip-advanced');
tooltipElements.forEach(function(elem) {
elem.addEventListener('mouseenter', function() {
var tooltipText = this.getAttribute('data-tooltip');
var tooltipSpan = document.createElement('span');
tooltipSpan.className = 'tooltiptext-advanced';
tooltipSpan.innerHTML = tooltipText;
this.appendChild(tooltipSpan);
setTimeout(function() {
tooltipSpan.style.visibility = 'visible';
tooltipSpan.style.opacity = 1;
}, 100);
});
elem.addEventListener('mouseleave', function() {
var tooltipSpan = this.querySelector('.tooltiptext-advanced');
if (tooltipSpan) {
tooltipSpan.style.visibility = 'hidden';
tooltipSpan.style.opacity = 0;
setTimeout(function() {
tooltipSpan.remove();
}, 300);
}
});
});
});
</script>
This script dynamically creates and displays tooltips when the user hovers over elements with the class tooltip-advanced
. The tooltip text is stored in the data-tooltip
attribute, which allows for easy customization. The tooltip is created and appended to the parent element when the mouseenter
event is triggered, and it is removed when the mouseleave
event occurs.
Styling and Positioning Tooltips
To improve the appearance and positioning of tooltips, you can use additional CSS properties and JavaScript logic. Here are some techniques to enhance tooltips:
Customizing Tooltip Styles
You can customize the tooltip's appearance by modifying the CSS properties of the .tooltiptext
or .tooltiptext-advanced
class. For example, you can change the background color, text color, font size, padding, and border radius:
<style>
.tooltiptext-advanced {
background-color: #333;
color: #fff;
font-size: 14px;
padding: 8px;
border-radius: 4px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
</style>
Positioning Tooltips
By default, the tooltip is positioned above the parent element. You can adjust the tooltip's position using CSS or JavaScript. For example, you can position the tooltip below, to the left, or to the right of the parent element:
<style>
.tooltiptext-advanced.bottom {
bottom: auto;
top: 125%;
}
.tooltiptext-advanced.left {
left: auto;
right: 125%;
margin-left: 0;
margin-right: 10px;
}
.tooltiptext-advanced.right {
left: 125%;
margin-left: 10px;
}
</style>
To apply these styles, add the corresponding class to the tooltip element when it is created:
<script>
elem.addEventListener('mouseenter', function() {
var tooltipText = this.getAttribute('data-tooltip');
var tooltipSpan = document.createElement('span');
tooltipSpan.className = 'tooltiptext-advanced bottom'; // Add position class here
tooltipSpan.innerHTML = tooltipText;
this.appendChild(tooltipSpan);
setTimeout(function() {
tooltipSpan.style.visibility = 'visible';
tooltipSpan.style.opacity = 1;
}, 100);
});
</script>
Interactive Tooltips
Tooltips can be enhanced with interactivity, allowing users to interact with the tooltip content. This can be useful for displaying additional information, links, or forms. Here is an example of an interactive tooltip:
<style>
.tooltip-interactive {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip-interactive .tooltiptext-interactive {
visibility: hidden;
width: 200px;
background-color: #333;
color: #fff;
text-align: left;
border-radius: 4px;
padding: 10px;
position:
absolute;
z-index: 1;
bottom: 125%;
left: 50%;
margin-left: -100px;
opacity: 0;
transition: opacity 0.3s;
}
.tooltip-interactive:hover .tooltiptext-interactive {
visibility: visible;
opacity: 1;
}
.tooltip-interactive .tooltiptext-interactive a {
color: #ffd700;
}
</style>
<div class="tooltip-interactive">
Hover over me
<div class="tooltiptext-interactive">
<p>Here is some interactive content.</p>
<p><a href="#">Click here</a> for more information.</p>
</div>
</div>
This example demonstrates an interactive tooltip containing text and a link. The tooltip is styled using the .tooltip-interactive
and .tooltiptext-interactive
classes, and it becomes visible when the user hovers over the parent element.
Tooltip Libraries and Plugins
There are many libraries and plugins available that provide advanced tooltip functionality out of the box. These tools can save you time and effort by offering a wide range of features and customization options. Some popular tooltip libraries include:
- Popper.js: A powerful library for positioning tooltips and popovers.
- Tippy.js: A lightweight and flexible tooltip library.
- Tooltip.js: A simple tooltip library by HubSpot.
These libraries typically offer advanced features such as automatic positioning, theming, animations, and more. They are well-documented and easy to integrate into your projects.
Accessibility Considerations
When creating tooltips, it is important to consider accessibility to ensure that all users, including those with disabilities, can access the tooltip content. Here are some tips for making your tooltips more accessible:
- Keyboard Navigation: Ensure that tooltips can be triggered using keyboard navigation. This can be achieved by adding
tabindex="0"
to the parent element and handling thefocus
andblur
events to show and hide the tooltip. - ARIA Roles and Properties: Use ARIA roles and properties to provide additional information about the tooltip to screen readers. For example, use
role="tooltip"
andaria-describedby
to associate the tooltip with its parent element. - Content Accessibility: Ensure that the tooltip content is readable and understandable. Avoid using tooltips for critical information that must be accessible to all users.
Example of Accessible Tooltip
<style>
.tooltip-accessible {
position: relative;
display: inline-block;
cursor: pointer;
}
.tooltip-accessible .tooltiptext-accessible {
visibility: hidden;
width: 120px;
background-color: black;
color: #fff;
text-align: center;
border-radius: 6px;
padding: 5px 0;
position: absolute;
z-index: 1;
bottom: 125%;
left: 50%;
margin-left: -60px;
opacity: 0;
transition: opacity 0.3s;
}
.tooltip-accessible:focus .tooltiptext-accessible,
.tooltip-accessible:hover .tooltiptext-accessible {
visibility: visible;
opacity: 1;
}
</style>
<div class="tooltip-accessible" tabindex="0" aria-describedby="tooltip1">
Hover or focus on me
<span id="tooltip1" role="tooltip" class="tooltiptext-accessible">Accessible tooltip text</span>
</div>
This example demonstrates an accessible tooltip that can be triggered using both mouse hover and keyboard focus. The tooltip is associated with its parent element using the aria-describedby
attribute and the role="tooltip"
attribute.
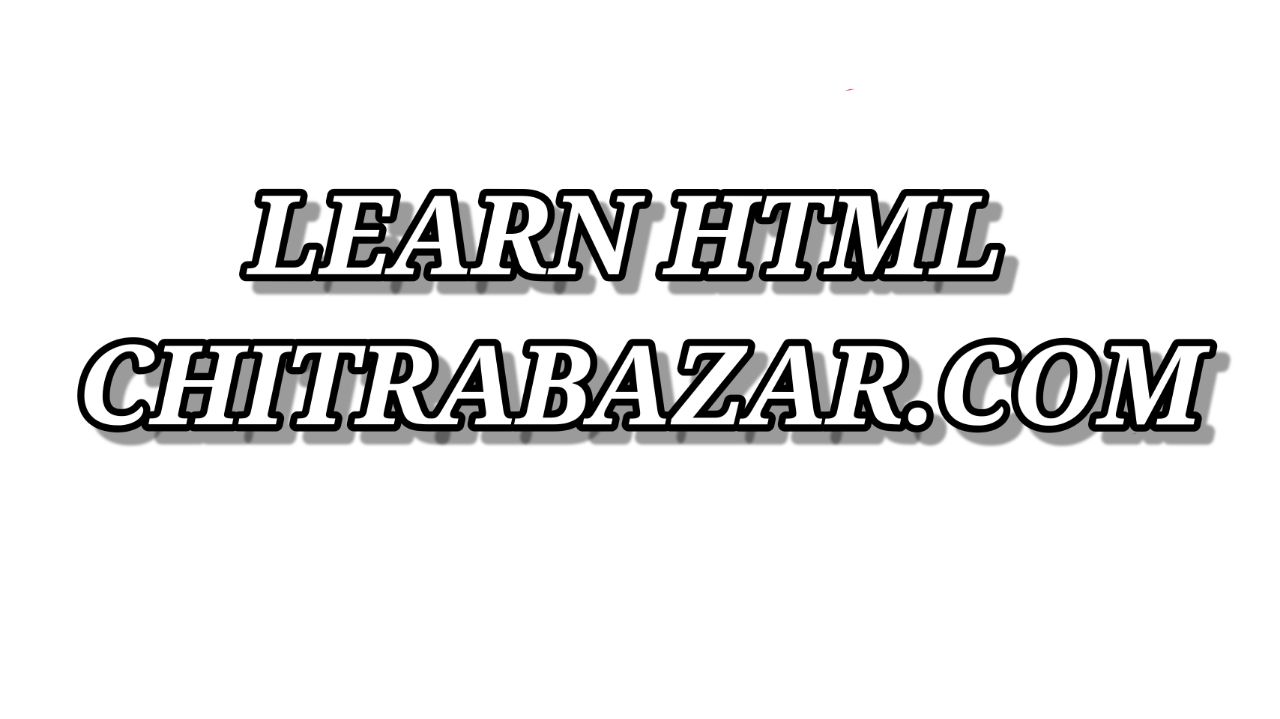
Tooltips are a valuable tool for enhancing the user experience by providing additional information and context without cluttering the interface. By using HTML, CSS, and JavaScript, you can create simple or advanced tooltips that are both functional and visually appealing. Additionally, consider accessibility to ensure that all users can benefit from your tooltips. With the right implementation and styling, tooltips can significantly improve the usability and interactivity of your web applications.