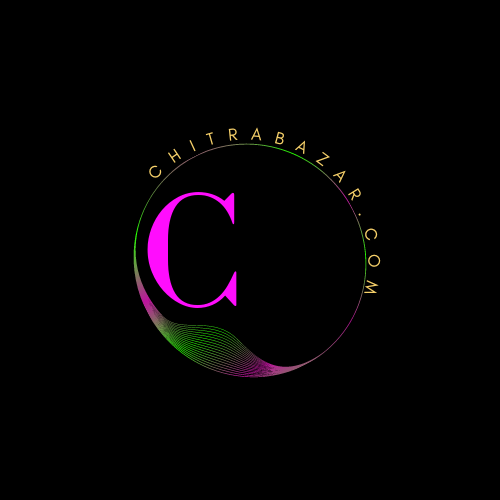
File Uploads
Overview of File uploads
File uploads are a common feature in web applications, allowing users to upload documents, images, videos, and other files. Implementing file uploads involves both front-end and back-end components. This guide covers the basics of creating a file upload form in HTML, handling file uploads on the server-side, and addressing common questions related to file uploads.
HTML Form for File Uploads
An HTML form with file input allows users to select files from their device and upload them to a server. The <input>
element with the type attribute set to "file" is used to create a file input field.
Basic HTML Structure
To create a basic file upload form, use the following HTML code:
Example
<form action="/upload" method="post" enctype="multipart/form-data">
<div class="form-group">
<label for="fileUpload">Choose file:</label>
<input type="file" id="fileUpload" name="fileUpload">
</div>
<button type="submit">Upload</button>
</form>
This form includes a file input field and a submit button. The enctype="multipart/form-data"
attribute is required for forms that include file uploads.
Handling File Uploads on the Server-Side
To handle file uploads, you need server-side code to process the uploaded files. The implementation depends on the server-side technology you are using. Below are examples for handling file uploads in PHP and Node.js.
PHP Example
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileUpload"]["name"]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($target_file, PATHINFO_EXTENSION));
// Check if file already exists
if (file_exists($target_file)) {
echo "Sorry, file already exists.";
$uploadOk = 0;
}
// Check file size
if ($_FILES["fileUpload"]["size"] > 500000) {
echo "Sorry, your file is too large.";
$uploadOk = 0;
}
// Allow certain file formats
if ($imageFileType != "jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif") {
echo "Sorry, only JPG, JPEG, PNG & GIF files are allowed.";
$uploadOk = 0;
}
// Check if $uploadOk is set to 0 by an error
if ($uploadOk == 0) {
echo "Sorry, your file was not uploaded.";
// if everything is ok, try to upload file
} else {
if (move_uploaded_file($_FILES["fileUpload"]["tmp_name"], $target_file)) {
echo "The file ". htmlspecialchars(basename($_FILES["fileUpload"]["name"])). " has been uploaded.";
} else {
echo "Sorry, there was an error uploading your file.";
}
}
}
?>
This PHP script handles file uploads by checking file size, file type, and whether the file already exists before saving the file to the server.
Node.js Example
const express = require('express');
const multer = require('multer');
const path = require('path');
const app = express();
const port = 3000;
const storage = multer.diskStorage({
destination: function (req, file, cb) {
cb(null, 'uploads/')
},
filename: function (req, file, cb) {
cb(null, file.fieldname + '-' + Date.now() + path.extname(file.originalname))
}
});
const upload = multer({ storage: storage });
app.post('/upload', upload.single('fileUpload'), (req, res) => {
res.send('File uploaded successfully!');
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
This Node.js example uses the Multer middleware to handle file uploads. The uploaded files are saved to the "uploads" directory with a unique filename.
Styling File Upload Inputs
File input fields can be styled to match the design of your website. Customizing file input fields often involves hiding the default input and creating a custom button.
Example
<style>
.file-upload-wrapper {
position: relative;
display: inline-block;
}
.file-upload-input {
position: absolute;
left: 0;
top: 0;
opacity: 0;
width: 100%;
height: 100%;
cursor: pointer;
}
.file-upload-button {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
border-radius: 4px;
}
.file-upload-button:hover {
background-color: #0056b3;
}
</style>
<form action="/upload" method="post" enctype="multipart/form-data">
<div class="file-upload-wrapper">
<button class="file-upload-button" type="button">Choose File</button>
<input type="file" class="file-upload-input" id="fileUpload" name="fileUpload">
</div>
<button type="submit">Upload</button>
</form>
This example hides the default file input and uses a button with custom styles. The hidden input is positioned over the button, allowing users to click the button to open the file picker.
Handling Multiple File Uploads
To allow users to upload multiple files at once, set the multiple
attribute on the file input element. The server-side code must also be adjusted to handle multiple files.
HTML Example
<form action="/upload" method="post" enctype="multipart/form-data">
<div class="form-group">
<label for="fileUpload">Choose files:</label>
<input type="file" id="fileUpload" name="fileUpload[]" multiple>
</div>
<button type="submit">Upload</button>
</form>
This form allows users to select multiple files for upload by adding the multiple
attribute to the file input.
PHP Example for Multiple File Uploads
<?php
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$total = count($_FILES['fileUpload']['name']);
for ($i = 0; $i < $total; $i++) {
$target_dir = "uploads/";
$target_file = $target_dir . basename($_FILES["fileUpload"]["name"][$i]);
$uploadOk = 1;
$imageFileType = strtolower(pathinfo($target_file, PATHINFO_EXTENSION));
// Check if file already exists
if (file_exists($target_file)) {
echo "Sorry, file already exists.";
$uploadOk = 0;
}
// Check file size
if ($_FILES["fileUpload"]["size"][$i] > 500000) {
echo "Sorry, your file is too large.";
$uploadOk = 0;
}
// Allow certain file formats
if ($imageFileType !=
"jpg" && $imageFileType != "png" && $imageFileType != "jpeg" && $imageFileType != "gif") {
echo "Sorry, only JPG, JPEG, PNG & GIF files are allowed.";
$uploadOk = 0;
}
// Check if $uploadOk is set to 0 by an error
if ($uploadOk == 0) {
echo "Sorry, your file was not uploaded.";
// if everything is ok, try to upload file
} else {
if (move_uploaded_file($_FILES["fileUpload"]["tmp_name"][$i], $target_file)) {
echo "The file ". htmlspecialchars(basename($_FILES["fileUpload"]["name"][$i])). " has been uploaded.";
} else {
echo "Sorry, there was an error uploading your file.";
}
}
}
}
?>
This PHP script processes multiple file uploads by iterating through the files array and handling each file individually.
Node.js Example for Multiple File Uploads
const express = require('express');
const multer = require('multer');
const path = require('path');
const app = express();
const port = 3000;
const storage = multer.diskStorage({
destination: function (req, file, cb) {
cb(null, 'uploads/')
},
filename: function (req, file, cb) {
cb(null, file.fieldname + '-' + Date.now() + path.extname(file.originalname))
}
});
const upload = multer({ storage: storage });
app.post('/upload', upload.array('fileUpload', 10), (req, res) => {
res.send('Files uploaded successfully!');
});
app.listen(port, () => {
console.log(`Server running on port ${port}`);
});
This Node.js example uses Multer to handle multiple file uploads. The upload.array
method is used to specify the file input name and the maximum number of files.
Security Considerations
When handling file uploads, it is crucial to implement security measures to prevent attacks such as file inclusion and denial of service. Here are some best practices:
- Validate the file type: Only allow specific file types (e.g., images, documents).
- Check the file size: Limit the maximum file size to prevent large file uploads.
- Rename uploaded files: Use unique filenames to avoid conflicts and obfuscate the original filename.
- Store files outside the web root: Prevent direct access to uploaded files by storing them outside the publicly accessible directory.
- Scan files for malware: Use antivirus software to scan uploaded files for potential threats.
Common Questions and Answers
Questions and Answers
To allow users to upload multiple files, add the multiple
attribute to the file input element. The server-side code must also be adjusted to handle multiple files.
You can limit the types of files that can be uploaded by checking the file extension or MIME type on the server side. Additionally, you can use the accept
attribute on the file input element to specify the allowed file types.
Example
<input type="file" id="fileUpload" name="fileUpload" accept=".jpg,.png,.gif">
In Node.js, you can use the Multer middleware to handle file uploads. Multer allows you to configure storage options and handle single or multiple file uploads.
To secure your file upload implementation, validate file types and sizes, rename uploaded files, store files outside the web root, and scan files for malware. Implementing these measures helps prevent various security threats.
You can style the file input field by hiding the default input and creating a custom button. Position the hidden input over the button to allow users to click the button to open the file picker.
Example
<style>
.file-upload-wrapper {
position: relative;
display: inline-block;
}
.file-upload-input {
position: absolute;
left: 0;
top: 0;
opacity: 0;
width: 100%;
height: 100%;
cursor: pointer;
}
.file-upload-button {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
border-radius: 4px;
}
.file-upload-button:hover {
background-color: #0056b3;
}
</style>
<form action="/upload" method="post" enctype="multipart/form-data">
<div class="file-upload-wrapper">
<button class="file-upload-button" type="button">Choose File</button>
<input type="file" class="file-upload-input" id="fileUpload" name="fileUpload">
</div>
<button type="submit">Upload</button>
</form>
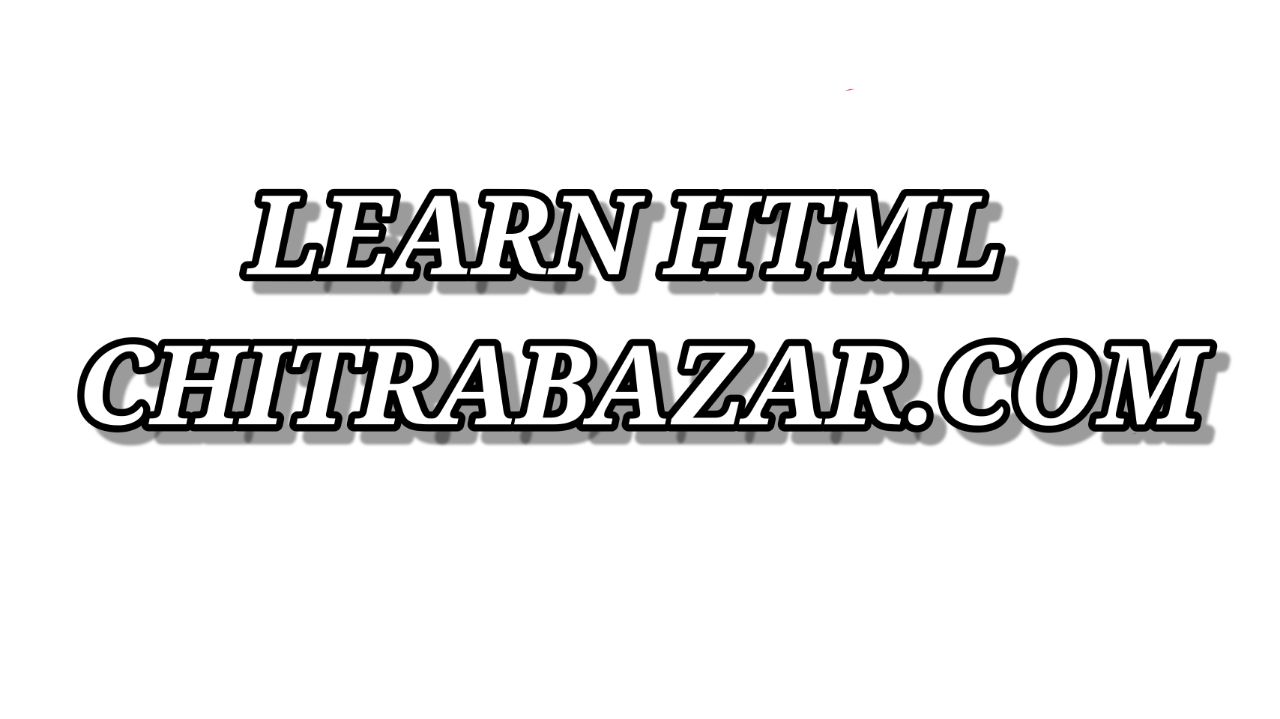
Note - File uploads are an essential feature in many web applications, allowing users to share and store files on the server. Understanding how to create file upload forms, handle file uploads on the server-side, style file inputs, and implement security measures is crucial for web developers. By following the guidelines and examples provided, you can implement secure and user-friendly file upload functionality in your web applications.