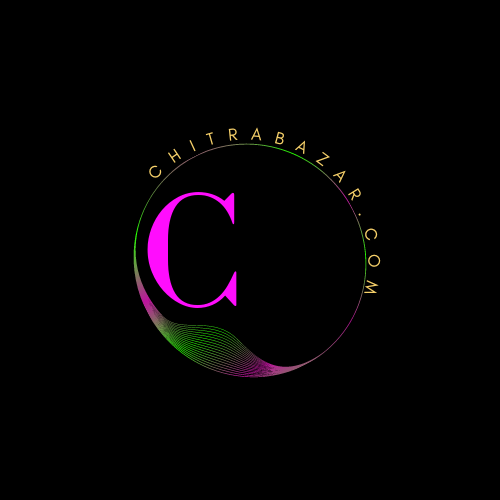
Form Validation
Introduction
Form validation is essential in web development to ensure that users submit valid and usable data. This guide provides a comprehensive overview of form validation, covering client-side and server-side validation, HTML attributes, JavaScript validation, and best practices. By the end of this guide, you will have a thorough understanding of how to implement robust form validation.
Client-Side Validation
Client-side validation occurs in the browser before the data is submitted to the server. This type of validation provides immediate feedback to users, improving the user experience. HTML5 provides built-in validation attributes that make it easy to implement client-side validation.
Required Attribute
The required
attribute ensures that the input field is not left empty.
<input type="text" name="username" required>
Pattern Attribute
The pattern
attribute specifies a regular expression that the input field’s value must match.
<input type="text" name="phone" pattern="\d{3}[\-]\d{3}[\-]\d{4}">
Email Validation
The type="email"
attribute ensures that the input is a valid email address.
<input type="email" name="email" required>
Number Validation
The type="number"
attribute is used to collect numerical input, with options to specify minimum and maximum values.
<input type="number" name="age" min="18" max="99" required>
Date Validation
The type="date"
attribute is used to collect date input from users.
<input type="date" name="birthday" required>
Example of HTML5 Validation
<form action="submit-form.php" method="post">
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<br>
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="18" max="99" required>
<br>
<input type="submit" value="Submit">
</form>
Server-Side Validation
Server-side validation occurs on the server after the data has been submitted. This type of validation is crucial for security, as it prevents malicious data from being processed by the server.
PHP Example
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$username = clean_input($_POST["username"]);
$email = clean_input($_POST["email"]);
$age = clean_input($_POST["age"]);
if (empty($username)) {
$nameErr = "Name is required";
}
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$emailErr = "Invalid email format";
}
if (!filter_var($age, FILTER_VALIDATE_INT) || $age < 18 || $age > 99) {
$ageErr = "Invalid age";
}
}
function clean_input($data) {
$data = trim($data);
$data = stripslashes($data);
$data = htmlspecialchars($data);
return $data;
}
?>
JavaScript Validation
JavaScript can be used to create custom validation rules and provide immediate feedback to users. Below is an example of JavaScript validation:
JavaScript Validation Example
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Validation Example</title>
<script>
function validateForm() {
var username = document.forms["myForm"]["username"].value;
var email = document.forms["myForm"]["email"].value;
var age = document.forms["myForm"]["age"].value;
var emailPattern = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (username == "") {
alert("Name must be filled out");
return false;
}
if (!emailPattern.test(email)) {
alert("Invalid email format");
return false;
}
if (isNaN(age) || age < 18 || age > 99) {
alert("Invalid age");
return false;
}
return true;
}
</script>
</head>
<body>
<form name="myForm" onsubmit="return validateForm()" method="post">
Name: <input type="text" name="username">
<br>
Email: <input type="email" name="email">
<br>
Age: <input type="number" name="age">
<br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Common Validation Techniques
There are various techniques for validating form inputs. Here are some common methods:
- Required Field Validation: Ensures that the field is not empty.
- Length Check: Ensures that the input meets the minimum or maximum length requirements.
- Range Check: Ensures that the numerical input falls within a specified range.
- Format Check: Ensures that the input matches a specific format, such as an email address or phone number.
Regular Expressions for Validation
Regular expressions are patterns used to match character combinations in strings. They are useful for validating the format of inputs.
Regular Expression Examples
Validation | Regular Expression |
---|---|
/^[^\s@]+@[^\s@]+\.[^\s@]+$/ |
|
Phone Number (US) | /^\d{3}[\-]\d{3}[\-]\d{4}$/ |
Postal Code (US) | /^\d{5}(?:[-\s]\d{4})?$/ |
Best Practices for Form Validation
Following best practices ensures your forms are secure, user-friendly, and accessible:
- Always validate input on both the client and server sides.
- Provide clear and concise error messages to guide users.
- Use semantic HTML elements to improve accessibility.
- Group related fields using
<fieldset>
and<legend>
elements. - Ensure your forms are responsive and work well on all devices.
- Use placeholders to provide examples of the expected input.
- Consider the privacy and security of user data, using HTTPS to encrypt form submissions.
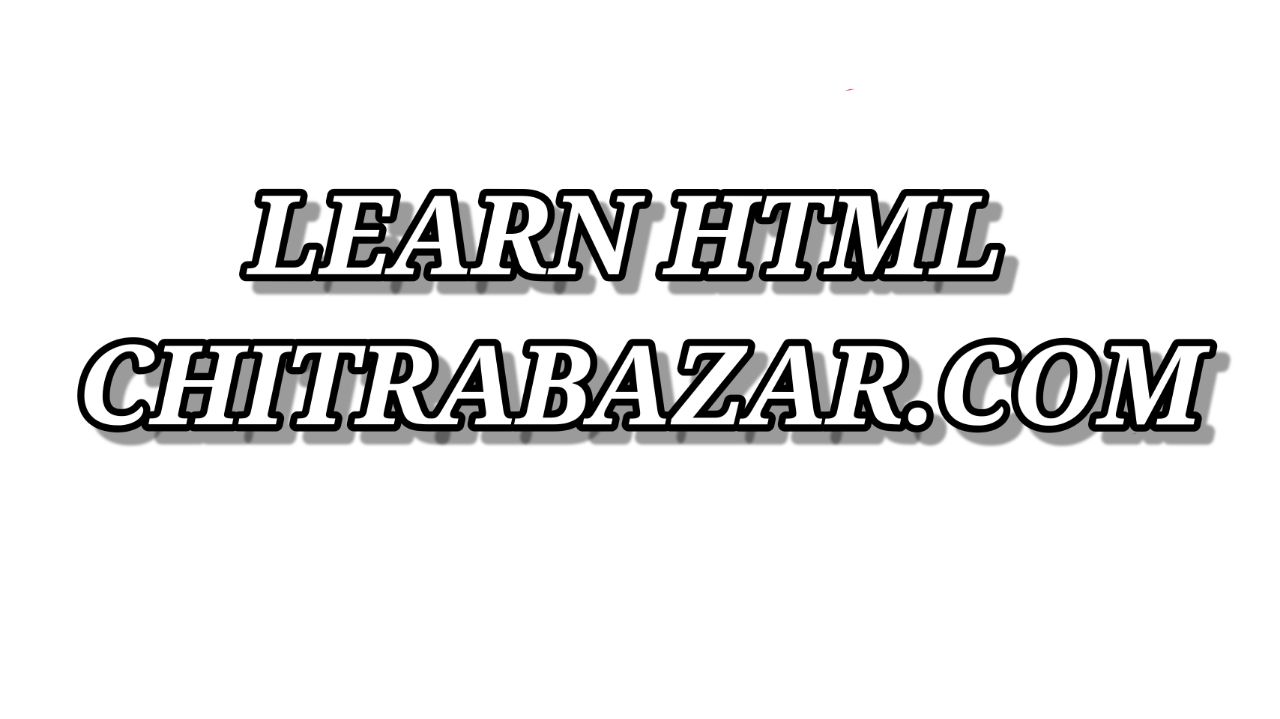