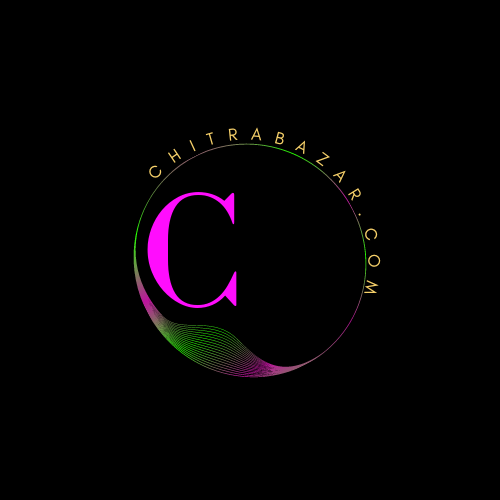
Forms and Input Elements in HTML
Forms in HTML
The <form>
element is a container for different types of input elements. It is used to collect user inputs and send them to a server for processing.
Basic Form Structure
<form action="submit_form.php" method="post">
<!-- Form elements go here -->
</form>
Attributes of the <form>
element:
action
: Specifies the URL to which the form data will be sent.method
: Specifies the HTTP method to be used when sending form data. Common values areget
andpost
.
Input Elements
Input elements are the building blocks of a form. They allow users to enter data and interact with the form. The <input>
element is the most commonly used input element.
Text Input
A text input allows the user to enter a single line of text.
<input type="text" name="username" placeholder="Enter your username">
Password Input
A password input masks the entered text, typically used for passwords.
<input type="password" name="password" placeholder="Enter your password">
Email Input
An email input validates that the entered text is in the format of an email address.
<input type="email" name="email" placeholder="Enter your email">
Number Input
A number input allows the user to enter numerical values.
<input type="number" name="age" min="1" max="100">
Date Input
A date input allows the user to select a date from a date picker.
<input type="date" name="birthday">
Radio Button
Radio buttons allow the user to select one option from a set of options.
<input type="radio" name="gender" value="male"> Male
<input type="radio" name="gender" value="female"> Female
Checkbox
Checkboxes allow the user to select one or more options from a set of options.
<input type="checkbox" name="interests" value="sports"> Sports
<input type="checkbox" name="interests" value="music"> Music
Submit Button
A submit button sends the form data to the server.
<input type="submit" value="Submit">
Textarea Element
The <textarea>
element is used for multi-line text input.
<textarea name="message" rows="4" cols="50" placeholder="Enter your message"></textarea>
Select Element
The <select>
element creates a drop-down list.
<select name="country">
<option value="usa">USA</option>
<option value="canada">Canada</option>
<option value="uk">UK</option>
</select>
Form Validation
HTML5 introduces built-in form validation. You can use attributes like required
, pattern
, min
, max
, minlength
, and maxlength
to validate user inputs.
Required Field
The required
attribute specifies that an input field must be filled out before submitting the form.
<input type="text" name="username" required>
Pattern Validation
The pattern
attribute specifies a regular expression that the input field's value must match.
<input type="text" name="phone" pattern="[0-9]{3}-[0-9]{3}-[0-9]{4}" placeholder="123-456-7890">
Accessibility Considerations
Ensuring forms are accessible is crucial for usability. Use the following practices:
- Label each input element with the
<label>
element. - Use the
for
attribute in labels to associate them with input elements.
<label for="username">Username:</label>
<input type="text" id="username" name="username">
Form Examples
Contact Form
<form action="contact_form.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required><br><br>
<label for="message">Message:</label><br>
<textarea id="message" name="message" rows="4" cols="50" required></textarea><br><br>
<input type="submit" value="Send">
</form>
Survey Form
<form action="survey_form.php" method="post">
<p>How did you hear about us?</p>
<input type="radio" id="friends" name="source" value="friends">
<label for="friends">Friends</label><br>
<input type="radio" id="advertisement" name="source" value="advertisement">
<label for="advertisement">Advertisement</label><br>
<input type="radio" id="other" name="source" value="other">
<label for="other">Other</label><br><br>
<label for="satisfaction">Rate your satisfaction:</label>
<input type="range" id="satisfaction" name="satisfaction" min="1" max="10"><br><br>
<input type="submit" value="Submit">
</form>
Example of Forms and Input Elements in HTML
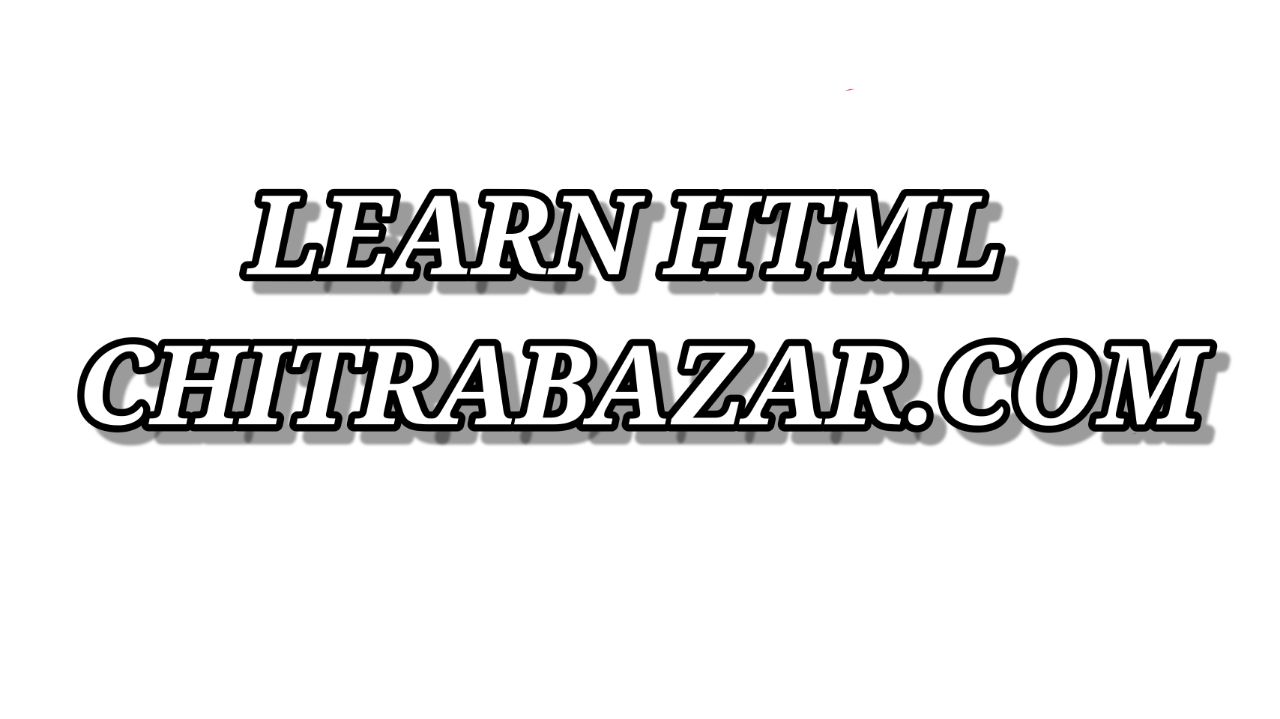