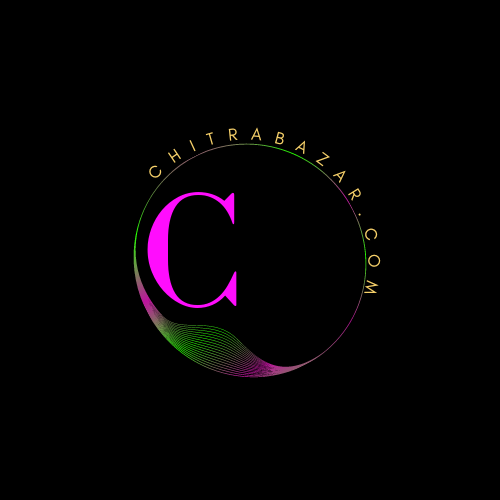
HTML Forms
Introduction
HTML forms are a crucial element of web development, allowing users to submit data to a web server. This guide provides a comprehensive overview of HTML forms, including their structure, input types, attributes, validation, and best practices. By the end of this guide, you will have a thorough understanding of how to create and manage HTML forms effectively.
Form Structure
The basic structure of an HTML form involves the <form>
element, which contains various input elements and other form controls. The <form>
element requires at least two attributes: action
and method
.
Basic Form Structure
<form action="submit-form.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<br>
<input type="submit" value="Submit">
</form>
Form Attributes
Attributes provide additional information about form elements, enhancing their functionality. Some of the key attributes include:
Attribute | Description | Example |
---|---|---|
action |
Specifies the URL where the form data will be sent for processing. | action="submit-form.php" |
method |
Defines the HTTP method to be used when sending form data. Common values are get and post . |
method="post" |
enctype |
Specifies the encoding type of the form data when using the POST method. Common values are application/x-www-form-urlencoded and multipart/form-data . |
enctype="multipart/form-data" |
autocomplete |
Enables or disables autocomplete for form fields. | autocomplete="on" |
target |
Specifies where to display the response after submitting the form. | target="_blank" |
Input Types
HTML provides a variety of input types to collect different kinds of data from users. Below are some of the most commonly used input types:
Text Input
Used to collect single-line text input from users.
<input type="text" name="username">
Password Input
Used to collect password input, which obscures the characters entered by the user.
<input type="password" name="password">
Email Input
Used to collect email addresses and provides basic validation.
<input type="email" name="email">
Number Input
Used to collect numerical input, with options to specify minimum and maximum values.
<input type="number" name="age" min="1" max="100">
Date Input
Used to collect date input from users.
<input type="date" name="birthday">
Radio Buttons
Used to collect one option from a set of predefined options.
<input type="radio" name="gender" value="male"> Male
<input type="radio" name="gender" value="female"> Female
Checkboxes
Used to collect multiple options from a set of predefined options.
<input type="checkbox" name="interests" value="coding"> Coding
<input type="checkbox" name="interests" value="music"> Music
<input type="checkbox" name="interests" value="sports"> Sports
Select Dropdown
Used to collect one option from a dropdown list.
<select name="country">
<option value="usa">USA</option>
<option value="canada">Canada</option>
<option value="uk">UK</option>
</select>
Textarea
Used to collect multi-line text input from users.
<textarea name="message" rows="4" cols="50"></textarea>
File Input
Used to allow users to upload files.
<input type="file" name="upload">
Submit Button
Used to submit the form data to the server.
<input type="submit" value="Submit">
Form Validation
Form validation ensures that users provide the necessary information in the correct format. HTML5 provides built-in validation features:
Required Attribute
Ensures that the input field is not left empty.
<input type="text" name="username" required>
Pattern Attribute
Specifies a regular expression that the input field’s value must match.
<input type="text" name="phone" pattern="\d{3}[\-]\d{3}[\-]\d{4}">
Min and Max Attributes
Specifies the minimum and maximum values for numerical input fields.
<input type="number" name="age" min="18" max="99">
Email Validation
Ensures that the input is a valid email address.
<input type="email" name="email" required>
Custom Validation
JavaScript can be used to create custom validation rules for more complex scenarios.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Custom Validation Example</title>
<script>
function validateForm() {
var x = document.forms["myForm"]["username"].value;
if (x == "") {
alert("Name must be filled out");
return false;
}
}
</script>
</head>
<body>
<form name="myForm" onsubmit="return validateForm()" method="post">
Name: <input type="text" name="username">
<br>
Email: <input type="email" name="email">
<br>
<input type="submit" value="Submit">
</form>
</body>
</html>
Best Practices for HTML Forms
Following best practices ensures that your forms are user-friendly, accessible, and secure:
- Use semantic HTML elements (e.g.,
<label>
,<fieldset>
,<legend>
) to improve accessibility. - Provide clear and concise labels for each form element.
- Group related form elements using the
<fieldset>
element. - Use placeholder text to provide examples of the expected input.
- Validate form data on both the client side (using HTML5 validation attributes and JavaScript) and the server side to ensure security.
- Ensure your forms are responsive and work well on all devices.
- Use the
<button>
element for buttons instead of<input type="button">
for more flexibility and accessibility. - Consider the privacy and security of user data, using HTTPS to encrypt form submissions.
Responsive Forms
Responsive forms adjust their layout based on the screen size. CSS media queries can be used to create responsive forms:
Example of a Responsive Form
<style>
body {
font-family: Arial, sans-serif;
}
.form-container {
width: 100%;
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ddd;
border-radius: 4px;
background-color: #f4f4f4;
}
@media (max-width: 600px) {
.form-container {
padding: 10px;
}
}
</style>
<div class="form-container">
<form action="submit-form.php" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<br>
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<br>
<input type="submit" value="Submit">
</form>
</div>
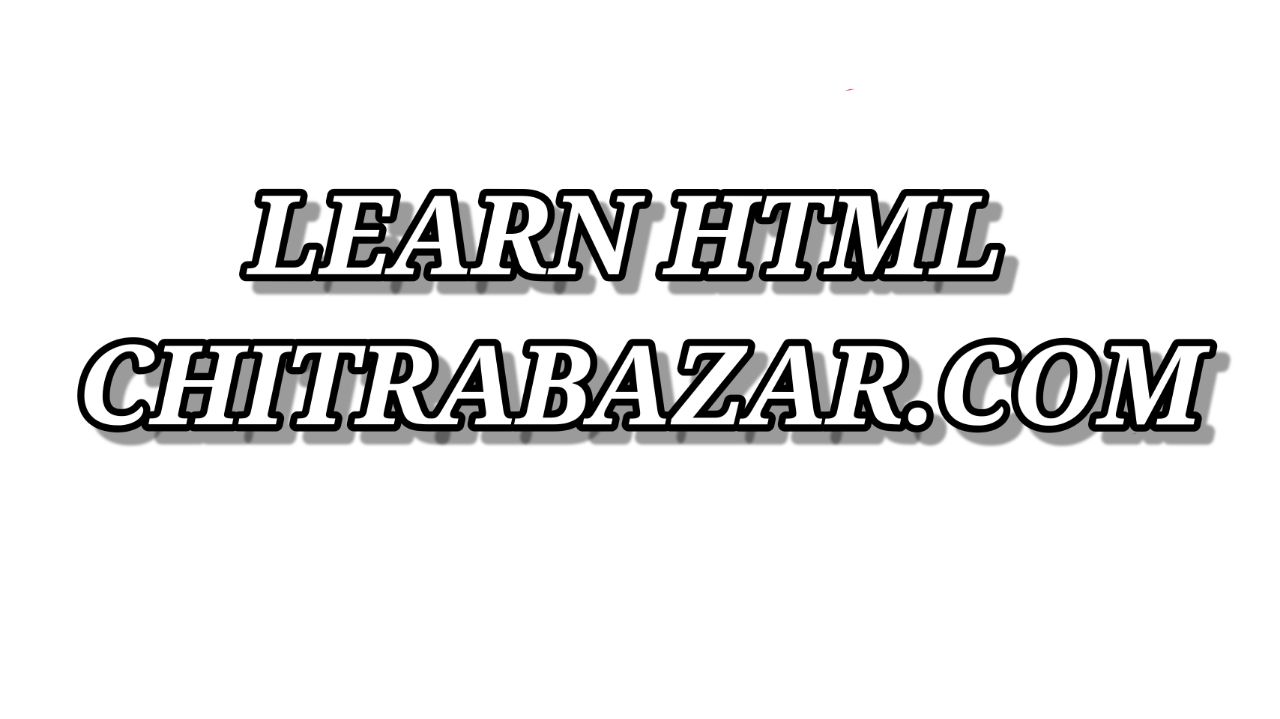