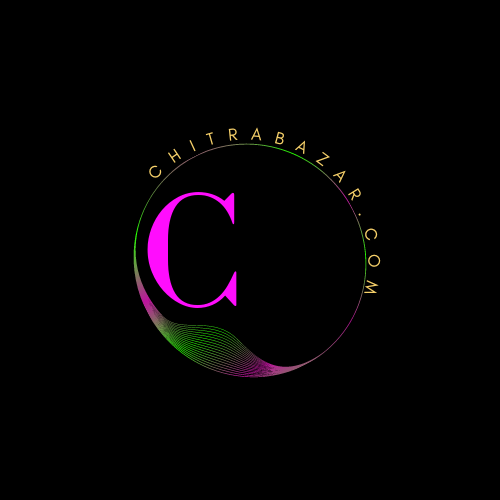
HTML5 Canvas
Learn about HTML5 Canvas
The HTML5 <canvas>
element is a powerful and versatile tool for rendering graphics on the web. It allows for dynamic, scriptable rendering of 2D shapes and bitmap images. This guide will explore the intricacies of working with the HTML5 Canvas API, covering everything from basic setup to advanced techniques.
Getting Started with Canvas
The <canvas>
element itself is quite simple. It only has two attributes, width
and height
, which define the size of the canvas. The default size is 300 pixels wide and 150 pixels high.
<canvas id="myCanvas" width="500" height="400">
Your browser does not support the HTML5 canvas tag.
</canvas>
The <canvas>
element above creates a 500x400 pixels drawing area. If the browser does not support the canvas element, it will display the fallback content: "Your browser does not support the HTML5 canvas tag."
Accessing the Canvas Context
To draw on the canvas, you need to access its drawing context. The getContext()
method returns an object that provides methods and properties for drawing on the canvas.
<script>
var canvas = document.getElementById('myCanvas');
var ctx = canvas.getContext('2d');
</script>
This script retrieves the canvas element by its ID and then gets the 2D drawing context, which is stored in the ctx
variable.
Drawing Shapes
With the 2D context, you can draw a variety of shapes. Let's start with some basic shapes:
Rectangles
The Canvas API provides several methods to draw rectangles:
fillRect(x, y, width, height)
: Draws a filled rectangle.strokeRect(x, y, width, height)
: Draws the outline of a rectangle.clearRect(x, y, width, height)
: Clears the specified area, making it fully transparent.
<script>
ctx.fillStyle = 'green';
ctx.fillRect(10, 10, 150, 100);
ctx.strokeStyle = 'red';
ctx.lineWidth = 5;
ctx.strokeRect(200, 10, 150, 100);
ctx.clearRect(220, 20, 50, 30);
</script>
This script draws a green filled rectangle, a red outlined rectangle with a 5-pixel border, and then clears a part of the outlined rectangle.
Paths and Lines
You can draw more complex shapes using paths. A path is a series of points connected by lines or curves. Here’s how to draw a triangle:
<script>
ctx.beginPath();
ctx.moveTo(75, 50);
ctx.lineTo(100, 75);
ctx.lineTo(100, 25);
ctx.closePath();
ctx.stroke();
</script>
The beginPath()
method starts a new path. The moveTo(x, y)
method moves the pen to the specified coordinates, and the lineTo(x, y)
method draws a line from the current position to the specified coordinates. The closePath()
method closes the path, connecting the last point to the first. Finally, the stroke()
method renders the path.
Arcs and Circles
To draw arcs or circles, use the arc(x, y, radius, startAngle, endAngle, counterclockwise)
method:
<script>
ctx.beginPath();
ctx.arc(75, 75, 50, 0, Math.PI * 2, false);
ctx.stroke();
</script>
This script draws a circle with a radius of 50 pixels, centered at (75, 75). The arc starts at angle 0 and ends at angle 2π (a full circle).
Working with Colors and Styles
The Canvas API allows you to style your drawings using colors, gradients, and patterns.
Colors
Set the color for shapes and lines using the fillStyle
and strokeStyle
properties:
<script>
ctx.fillStyle = 'blue';
ctx.fillRect(10, 10, 100, 100);
ctx.strokeStyle = 'red';
ctx.lineWidth = 5;
ctx.strokeRect(150, 10, 100, 100);
</script>
Gradients
You can create gradients using the createLinearGradient(x0, y0, x1, y1)
and createRadialGradient(x0, y0, r0, x1, y1, r1)
methods:
<script>
var linearGradient = ctx.createLinearGradient(0, 0, 200, 0);
linearGradient.addColorStop(0, 'blue');
linearGradient.addColorStop(1, 'green');
ctx.fillStyle = linearGradient;
ctx.fillRect(10, 10, 200, 100);
</script>
This script creates a linear gradient from blue to green and uses it to fill a rectangle.
Patterns
To use images as patterns, use the createPattern(image, repetition)
method:
<script>
var img = new Image();
img.onload = function() {
var pattern = ctx.createPattern(img, 'repeat');
ctx.fillStyle = pattern;
ctx.fillRect(0, 0, 300, 300);
};
img.src = 'path/to/image.png';
</script>
This script creates a repeating pattern from an image and uses it to fill a rectangle.
Transformations
The Canvas API supports various transformations such as translation, rotation, and scaling.
Translation
The translate(x, y)
method moves the canvas and its origin to a different point in the grid:
<script>
ctx.translate(50, 50);
ctx.fillStyle = 'blue';
ctx.fillRect(0, 0, 100, 100);
</script>
This script moves the canvas origin 50 pixels to the right and 50 pixels down, then draws a blue rectangle at the new origin.
Rotation
The rotate(angle)
method rotates the canvas around the current origin:
<script>
ctx.translate(100, 100);
ctx.rotate(Math.PI / 4); // Rotate 45 degrees
ctx.fillStyle = 'green';
ctx.fillRect(-50, -50, 100, 100);
</script>
This script moves the origin to (100, 100), rotates the canvas 45 degrees, and then draws a green square centered at the new origin.
Scaling
The scale(x, y)
method scales the canvas units by the factors specified along the X and Y axes:
<script>
ctx.scale(2, 2);
ctx.fillStyle = 'purple';
ctx.fillRect(10, 10, 50, 50);
</script>
This script scales the canvas units by a factor of 2, then draws a purple rectangle that appears twice as large.
Animating with Canvas
Animating on
the canvas involves clearing and redrawing the scene at regular intervals. The requestAnimationFrame()
method is commonly used for this purpose, as it provides a more efficient and accurate timing mechanism than setInterval()
or setTimeout()
.
<script>
var x = 0;
function draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = 'red';
ctx.fillRect(x, 50, 50, 50);
x += 1;
requestAnimationFrame(draw);
}
draw();
</script>
This script animates a red square moving horizontally across the canvas by clearing and redrawing the square at increasing X coordinates.
Working with Text
The Canvas API provides methods for drawing text. You can customize the font, size, and style of the text.
Drawing Text
The fillText(text, x, y)
and strokeText(text, x, y)
methods draw filled and outlined text respectively:
<script>
ctx.font = '30px Arial';
ctx.fillStyle = 'black';
ctx.fillText('Hello Canvas', 10, 50);
ctx.strokeStyle = 'blue';
ctx.strokeText('Hello Canvas', 10, 100);
</script>
This script draws "Hello Canvas" in black filled text and blue outlined text at specified positions.
Text Alignment
You can align text horizontally and vertically using the textAlign
and textBaseline
properties:
<script>
ctx.textAlign = 'center';
ctx.textBaseline = 'middle';
ctx.font = '30px Arial';
ctx.fillStyle = 'purple';
ctx.fillText('Centered Text', canvas.width / 2, canvas.height / 2);
</script>
This script centers the text both horizontally and vertically on the canvas.
Image Manipulation
The Canvas API can also manipulate images, including drawing images on the canvas, cropping them, and accessing pixel data.
Drawing Images
Use the drawImage()
method to draw images on the canvas:
<script>
var img = new Image();
img.onload = function() {
ctx.drawImage(img, 10, 10);
};
img.src = 'path/to/image.png';
</script>
This script draws an image at the specified position once it has loaded.
Cropping Images
You can also draw a cropped section of an image using an extended version of the drawImage()
method:
<script>
img.onload = function() {
ctx.drawImage(img, 50, 50, 100, 100, 10, 10, 100, 100);
};
</script>
This script draws a 100x100 pixel section of the image, starting at (50, 50), to a 100x100 area on the canvas at (10, 10).
Accessing Pixel Data
The getImageData(x, y, width, height)
method retrieves pixel data from the canvas:
<script>
var imageData = ctx.getImageData(0, 0, canvas.width, canvas.height);
var data = imageData.data;
for (var i = 0; i < data.length; i += 4) {
data[i] = 255 - data[i]; // Red
data[i + 1] = 255 - data[i + 1]; // Green
data[i + 2] = 255 - data[i + 2]; // Blue
}
ctx.putImageData(imageData, 0, 0);
</script>
This script inverts the colors of the entire canvas by manipulating the pixel data directly.
The HTML5 <canvas>
element is a powerful tool for creating dynamic graphics on the web. From drawing basic shapes and text to creating complex animations and manipulating images, the Canvas API provides a wide range of capabilities for web developers. Understanding and leveraging these features can significantly enhance the interactivity and visual appeal of web applications.